Note
Click here to download the full example code
Fusion3D exampleΒΆ
Fusion3D (mesh + texture from a volume) in Anatomist
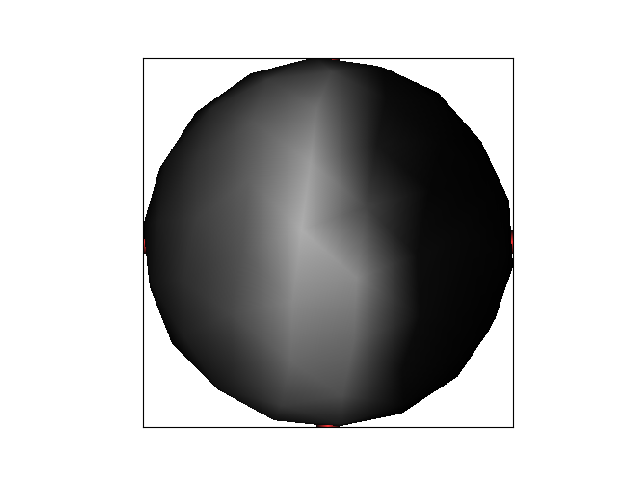
from __future__ import absolute_import
import anatomist.api as anatomist
# to expand the mesh bigger
from soma import aims
from soma.qt_gui.qt_backend import Qt
import numpy as np
import sys
runloop = False
if Qt.QApplication.instance() is None:
runloop = True
# initialize Anatomist
a = anatomist.Anatomist()
# load a volume in anatomist
avol = a.loadObject('irm.ima')
amesh = a.loadObject('test.mesh')
# this is to make the mesh bigger compared to the volume size
tr = aims.AffineTransformation3d()
tr.fromMatrix(np.matrix([[8., 0, 0, 204.4],
[0, 8., 0, 132.5],
[0, 0, 8., 68.4]]))
aims.SurfaceManip.meshTransform(amesh.toAimsObject(), tr)
amesh.UpdateMinAndMax()
# fusion the objects
fusion = a.fusionObjects(objects=[avol, amesh], method="Fusion3DMethod")
# params of the fusion
a.execute("Fusion3DParams", object=fusion,
method="line", submethod="mean", depth=4, step=1)
# open a window
win = a.createWindow('Axial')
# put volume in window
a.addObjects([fusion], [win])
# export the fusion texture in a file.
fusion.exportTexture("fusion.tex")
# display in matplotlib for sphinx_gallery
import matplotlib
matplotlib.use('agg', force=True) # force agg
win.imshow(show=True)
if runloop and 'sphinx_gallery' not in sys.modules:
Qt.QApplication.instance().exec_()
if runloop or 'sphinx_gallery' in sys.modules:
del fusion, win, amesh, avol, tr
Total running time of the script: ( 0 minutes 0.798 seconds)