brainvisa processing modules¶
brainvisa.axon¶
This module enables to start Brainvisa in batch mode through a python script.
It is useful to write a Python script that uses Brainvisa. Usage:
>>> import brainvisa.axon
>>> brainvisa.axon.initializeProcesses()
Loading toolbox ...
Then, Brainvisa, its processes and databases are loaded and it can be used as if it were started in batch mode (brainvisa -b
).
At the end of your script, call a cleanup function. It would be called automatically at exit, but it is better to call it from the main thread:
>>> brainvisa.axon.cleanup()
- brainvisa.axon.processes.cleanup()[source]¶
Cleanup to be done at Brainvisa exiting. This function is registered in atexit.
- brainvisa.axon.processes.initializeProcesses()[source]¶
This method intends to retrieve a list of all existing types in the BrainVISA ontology, of all processes and databases. This replicates the job which is usually done at the very beginning when BrainVISA starts, but here no GUI is created.
The processes are available through functions in
brainvisa.processes
. The databases are inbrainvisa.data.neuroHierarchy.databases
. The types are available through functions inbrainvisa.data.neuroDiskItems
.
brainvisa.processes¶
This module contains classes defining Brainvisa processes and pipelines.
The main class in this module is Process
. It is the base class for all Brainvisa processes. It inherits from the class Parameterized
that defines an object with a list of parameters that we call a signature.
When the processes are loaded at Brainvisa startup, information about them is stored in ProcessInfo
objects.
- Several functions are available in this module to get information about the processes or to get instances of the processes:
- To modify, the lists of processes, several functions are also available:
- A pipeline is defined as a specific process that has an execution node that describes the pipeline structure. The base class for execution nodes is
ExecutionNode
. This class is specialized into several other classes, defining different types of pipelines: ProcessExecutionNode
: only one processSerialExecutionNode
: a list of execution nodes that have to be executed serially.ParallelExecutionNode
: a list of execution nodes that can be executed in parallel.SelectionExecutionNode
: a choice between several execution nodes.
- Specialized Process classes that use the different types of execution nodes also exist:
IterationProcess
: an iteration of a process on a set of data. Uses aParallelExecutionNode
.DistributedProcess
: a pipeline that have aParallelExecutionNode
SelectionProcess
: a pipeline that have aSelectionExecutionNode
.
As processes can be run in different contexts, an object representing this context is passed as a parameter in the processes execution function. This object is an intance of the class ExecutionContext
. A default context associated to the application also exists, to get it use the function defaultContext()
.
After loading, Brainvisa processes are stored in an object ProcessTrees
that represents the processes organization in toolboxes and categories. The function updatedMainProcessTree()
creates this object if it doesn’t exist yet and to returns it.
- Inheritance diagram:
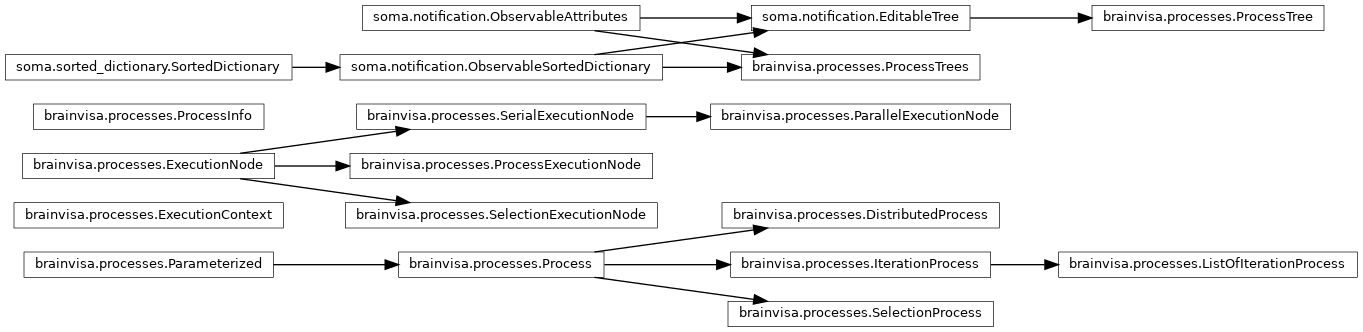
- Classes:
- class brainvisa.processes.Parameterized(signature)[source]¶
Bases:
object
This class represents an object that have a signature, that is to say a list of typed parameters.
A Parameterized object can notify the changes in its signature. The parameters can be linked together, that is to say, if the value of a parameter changes, the value of all linked parameters may change.
This object has an
initialization()
function that can define the links between the parameters and their initial values.- Attributes:
- signature¶
The signature is a
brainvisa.data.neuroData.Signature
. It contains the list of parameters accepted by the object and their types. The possible types are described inbrainvisa.data.neuroData
.
- signatureChangeNotifier¶
This variable is a
soma.notification.Notifier
. It calls its notify function when the signature of theParameterized
object changes.
- class brainvisa.processes.Process[source]¶
Bases:
Parameterized
This class represents a Brainvisa process or pipeline.
This object has a signature that describes its inputs and outputs and an execution function
execution()
. If it is a pipeline, it also have an execution node that describes the structure of the pipeline.- Attributes:
- signature¶
The signature is a
brainvisa.data.neuroData.Signature
. It contains the list of parameters accepted by the object and their types. The possible types are described inbrainvisa.data.neuroData
.
- category(string)¶
The processes are organized into categories. Generally, the category is the name of the directory where the process file is located.
- userLevel(integer)¶
The process is available in Brainvisa interface if its userLevel is lower or equal than the userLevel selected in Brainvisa options. 0 : Basic, 1: Advanced, 2: Expert.
- showMaximized(boolean)¶
If true, the process window is shown maximized with a frame around it.
- Methods:
- validation()[source]¶
This method can be overrideed in order to check if the process dependencies are available. It will be called at Brainvisa startup when the processes are loaded. If the method raises an exception, the process will not be available.
- setExecutionNode(eNode)[source]¶
Sets the execution node of the pipeline.
- Parameters:
eNode (
ExecutionNode
) – object that describes the structure of the pipeline.
- getAllParameters()[source]¶
Returns all the parameters of the current process and its children if it is a pipeline.
- Returns:
tuples (Parameterized, attribute name, attribute type)
- Return type:
generator
- allProcesses()[source]¶
Returns the current process and all its children if it is a pipeline.
- Return type:
generator
- pipelineStructure()[source]¶
Returns the description of a pipeline in a dictionary or the id of the process if it is a simple process.
- inlineGUI(values, context, parent, externalRunButton=False)[source]¶
This method can be overrideed in order to specialize buttons of the process window.
- Parameters:
context – the execution context of the process
parent – The parent widget
- Returns:
widget – the widget containing the buttons that will replace the default buttons (Run and Iterate)
- Return type:
QWidget
- _iterate(warn=True, **kwargs)[source]¶
Returns a list of copies of the current process with different parameters values.
- Parameters:
warn (bool) – raise an exception if iterated parameters lists sizes do not match
kwargs – dictionary containing a list of values for each parameter name. The first value is for the first process of the iteration and so on…
- class brainvisa.processes.ProcessInfo(id, name, signature, userLevel, category, fileName, roles, toolbox, module=None, showMaximized=False)[source]¶
Bases:
object
This object stores information about a process. Such objects are created at BrainVISA startup when the processes are loaded.
- id¶
Id of the process. It is the name of the file without extension in lowercase.
- name¶
Name of the process as it is displayed in the GUI.
- signature¶
Process excepted parameters.
- userLevel¶
User level needed to see the process.
- category¶
Process category path: <toolbox>/<category1>/<category2>/…
- showMaximized¶
Process window maximized state.
- fileName¶
Path to the file containing the source code of the process.
- roles¶
Tuple containing the specific roles of the process: viewer, converter, editor, importer.
- valid¶
False if the validation method of the process fails - default True.
- procdoc¶
The content of the .procdoc file associated to this process in a dictionary. It represents the documentation of the process.
- toolbox¶
The id of the toolbox containing the process.
- module¶
Module path to the source of the process related to the toolbox directory. <processes>.<category1>…<process>
- class brainvisa.processes.ExecutionNode(name='', optional=False, selected=True, guiOnly=False, parameterized=None, expandedInGui=False)[source]¶
Bases:
object
Base class for the classes that describe a pipeline structure.
- Parameters:
name (str) – name of the node - default ‘’.
optional (boolean) – indicates if this node is optional in the pipeline - default False.
selected (boolean) – indicates if the node is selected in the pipeline - default True.
guiOnly (boolean) – default False.
parameterized –
Parameterized
containing the signature of the node - default None.
- addChild(name, node, index=None)[source]¶
Add a new child execution node.
- Parameters:
name (string) – name which identifies the node
node – an
ExecutionNode
which will be added to this node’s children.
- addDoubleLink(destination, source, function=None)[source]¶
Creates a double link source -> destination and destination -> source.
- addExecutionDependencies(deps)[source]¶
Adds to the execution node dependencies on the execution of other nodes. This allows to build a dependencies structure which is not forced to be a tree, but can be a grapĥ. Dependencies are used to build Soma-Workflow workflows with correct dependencies.
- addLink(destination, source, function=None, destDefaultUpdate=True)[source]¶
Adds a parameter link like
Parameterized.addLink()
.
- allParameterFiles()[source]¶
Get recursively all parameters which are DiskItems, descending through the pipeline structure.
- property parameterized¶
The Parameterized object associated with this node.
The ExecutionNode keeps only a weak reference in _parameterized. This property returns a strong reference to the Parameterized object if it is still alive, otherwise it returns None.
- parent_pipeline()[source]¶
Returns the root pipeline process from which this node is part of, if it is actually part of a pipeline (otherwise None is returned).
New in Axon 4.6.
- parseParameterString(parameterString)[source]¶
Returns a tuple containing the
Parameterized
object of the child node indicated in the parameter string and the name of the parameter. May return None if the node is invalid.- Parameters:
parameterString (str) – references a parameter of a child node with a path like <node name 1>.<node name 2>…<parameter name>
- removeChild(name)[source]¶
Remove child execution node.
- Parameters:
name (string) – name which identifies the node
- removeDoubleLink(destination, source, function=None, show_warnings=True)[source]¶
Removes a double link source -> destination and destination -> source.
- class brainvisa.processes.ProcessExecutionNode(process, optional=False, selected=True, guiOnly=False, expandedInGui=False, altname=None, skip_invalid=False)[source]¶
Bases:
ExecutionNode
An execution node that has no children and run one process
- Parameters:
process (process id or instance or class) –
optional (bool) – may be unchecked in the pipeline (not run)
selected (bool) – checked in the pipeline, will be the selected one in a selection node
guiOnly (bool) – will be skipped in a non-interactive environment
expandedInGui (bool) – in the tree widget
altname (str) – alternative name displayed in the GUI
skip_invalid (bool) – marks the node as “optional”: if the process cannot be instantiated, the pipeline construction will not fail, but the node will not be added. Links involving this node will be also skipped silently. This is useful to buils pipelines with several alternative nodes which may be unavailable in some contexts (due to missing dependencies or external software, typically)
- addChild(name, node, index=None)[source]¶
Add a new child execution node.
- Parameters:
name (string) – name which identifies the node
node – an
ExecutionNode
which will be added to this node’s children.
- class brainvisa.processes.SerialExecutionNode(name='', optional=False, selected=True, guiOnly=False, parameterized=None, stopOnError=True, expandedInGui=False, possibleChildrenProcesses=None, notify=False)[source]¶
Bases:
ExecutionNode
An execution node that run all its children sequentially
- Parameters:
name (str) – name of the node - default ‘’.
optional (boolean) – indicates if this node is optional in the pipeline - default False.
selected (boolean) – indicates if the node is selected in the pipeline - default True.
guiOnly (boolean) – default False.
parameterized –
Parameterized
containing the signature of the node - default None.
- addChild(name=None, node=None, index=None)[source]¶
Add a new child execution node.
- Parameters:
name (string) – name which identifies the node
node – an
ExecutionNode
which will be added to this node’s children.
- class brainvisa.processes.ParallelExecutionNode(name='', optional=False, selected=True, guiOnly=False, parameterized=None, stopOnError=True, expandedInGui=False, possibleChildrenProcesses=None, notify=False)[source]¶
Bases:
SerialExecutionNode
An execution node that run all its children in any order (and in parallel if possible)
- Parameters:
name (str) – name of the node - default ‘’.
optional (boolean) – indicates if this node is optional in the pipeline - default False.
selected (boolean) – indicates if the node is selected in the pipeline - default True.
guiOnly (boolean) – default False.
parameterized –
Parameterized
containing the signature of the node - default None.
- class brainvisa.processes.SelectionExecutionNode(*args, **kwargs)[source]¶
Bases:
ExecutionNode
An execution node that run one of its children
- Parameters:
name (str) – name of the node - default ‘’.
optional (boolean) – indicates if this node is optional in the pipeline - default False.
selected (boolean) – indicates if the node is selected in the pipeline - default True.
guiOnly (boolean) – default False.
parameterized –
Parameterized
containing the signature of the node - default None.
- class brainvisa.processes.IterationProcess(name, processes, base=None)[source]¶
Bases:
Process
This class represents a set of process instances that can be executed in parallel.
It is used to iterate the same process on a set of data.
- class brainvisa.processes.ListOfIterationProcess(name, processes)[source]¶
Bases:
IterationProcess
An IterationProcess which has on its main signature a list of the first element of each sub-process.
Used for viewers and editors of ListOf()
- class brainvisa.processes.DistributedProcess(name, processes)[source]¶
Bases:
Process
This class represents a set of process instances that can be executed in parallel.
- class brainvisa.processes.SelectionProcess(name, processes)[source]¶
Bases:
Process
This class represents a choice between a list of processes.
- class brainvisa.processes.ExecutionContext(userLevel=None, debug=None)[source]¶
Bases:
object
This object represents the execution context of the processes.
Indeed, a process can be started in different contexts :
The user starts the process by clicking on the Run button in the graphical interface.
The process is started via a script. It is possible to run brainvisa in batch mode (without any graphical interface) and to run a process via a python function : brainvisa.processes.defaultContext().runProcess(…).
The process is a converter, so it can be run automatically by BrainVISA when a conversion is needed for another process parameters.
The process is a viewer or an editor, it is run when the user clicks on the corresponding icon to view or edit another process parameter.
The interactions with the user are different according to the context. That’s why the context object offers several useful functions to interact with BrainVISA and to call system commands. Here are these functions :
write()
,warning()
,error()
: prints a message, either in the graphical process window (in GUI mode) or in the terminal (in batch mode).log()
: writes a message in the BrainVISA log file.temporary()
: creates a temporary file.system()
: calls a system command.runProcess()
: runs a BrainVISA process.checkInterruption()
: defines a breakpoint.
- ask(message, *buttons, **kwargs)[source]¶
This method asks a question to the user. The message is displayed and the user is invited to choose a value among the propositions. The method returns the index of the chosen value, beginning by 0. If the answer is not valid, the returned value is -1. Sometimes, when the process is called automatically (in batch mode), these calls to context.ask are ignored and return directly -1 without asking question.
Example
>>> if context.ask('Is the result ok ?', 'yes', 'no') == 1: >>> try_again()
- checkInterruption()[source]¶
This function is used to define breakpoints. When the process execution reach a breakpoint, the user can interrupt the process. There are 4 types of breakpoints automatically added :
before each system call (context.system)
after each system call (context.system)
before each sub-process call (context.runProcess)
after each sub-process call (context.runProcess)
To allow the user to interrupt the process at another place, you have to use the function context.checkInterruption. If the user has clicked on the Interrupt button while the process runs, it will stop when reaching the checkInterruption point.
- dialog(*args)[source]¶
This method is available only in a graphical context. Like ask, it is used to ask a question to the user, but the dialog interface is customisable. It is possible to add a signature to the dialog : fields that the user has to fill in.
Example
>>> dial = context.dialog(1, 'Enter a value', Signature('param', Number()), _t_('OK'), _t_('Cancel')) >>> dial.setValue('param', 0) >>> r = dial.call() >>> if r == 0: >>> v=dial.getValue('param')
- error(*messages)[source]¶
This method is used to print an error message. Like the above function, it adds some HTML tags to change the appearance of the message and calls
write()
function.
- getConverter(source, dest, checkUpdate=True)[source]¶
Gets a converter process which can convert the source diskitem from its format to the destination format.
- getProgressInfo(process, childrencount=None, parent=None)[source]¶
Get the progress info for a given process or execution node, or create one if none already exists. A regular process may call it.
The output is a tuple containing the ProgressInfo and the process itself, just in case the input process is in fact a ProgressInfo instance. A ProgressInfo has no hard reference in BrainVISA: when you don’t need it anymore, it is destroyed via Python reference counting, and is considered done 100% for its parent.
- Parameters:
childrencount – it is the number of children that the process will have, and is not the same as the own count of the process in itself, which is in addition to children (and independent), and specified when using the progress() method.
- log(*args, **kwargs)[source]¶
context.log(what, when=None, html=’’, children=[], icon=None)
This method is used to add a message to BrainVISA log. The first parameter what is the name of the entry in the log, the message to write is in the html parameter.
- progress(value=None, count=None, process=None)[source]¶
Set the progress information for the parent process or ProgressInfo instance, and output it using the context output mechanisms.
- Parameters:
value – is the progress value to set. If none, the value will not be changed, but the current status will be shown.
count – is the maximum value for the process own progress value (not taking children into account).
process – is either the calling process, or the ProgressInfo.
- pythonSystem(*args, **kwargs)[source]¶
Specialization of system() for calling Python scripts
The script passed as the first agrument is called through the Python interpreter (
sys.executable
). The script will be searched inPATH
and its full path will be passed to the interpreter, unless the first argument begins with-
(which allows using-c
or-m
),On Unix, this should have the same result as using system(), but on Windows the script may not be recognized as a Python script by the system, so needs this wrapping.
NOTE: this method will always use the same interpreter as BrainVISA (i.e.
sys.executable
), regardless of the shebang line in your Python script. Therefore, you should make sure that your script is compatible with both Python 2 and Python 3.
- runInteractiveProcess(callMeAtTheEnd, process, *args, **kwargs)[source]¶
Runs a process in a new thread and calls a callback function when the execution is finished.
- Parameters:
callMeAtTheEnd (function) – callback function which will be called the process execution is finished.
process – id of the process which will be run.
- runProcess(_process, *args, **kwargs)[source]¶
It is possible to call a sub-process in the current process by calling context.runProcess.
The first argument is the process identifier, which is either the filename wihtout extension of the process or its english name. The other arguments are the values of the process parameters. All mandatory argument must have a value. The function returns the value returned by the sub-process execution method.
Example
>>> context.runProcess('do_something', self.input, self.output, value=3.14)
In this example, the process do_something is called with self.input as the first paramter value, self.ouput as the second parameter value and 3.14 to the parameter named value.
- showException(beforeError='', afterError='', exceptionInfo=None)[source]¶
same as the global brainvisa.processing.neuroException.showException() but displays in the current context (the process output box for instance)
- showProgress(value, count=None)[source]¶
Output the given progress value. This is just the output method which is overriden in subclassed contexts.
Users should normally not call it directory, but use progress() instead.
- system(*args, **kwargs)[source]¶
This function is used to call system commands. It is very similar to functions like
os.system()
in Python andsystem()
in C. The main difference is the management of messages sent on standard output. These messages are intercepted and reported in BrainVISA interface according to the current execution context.Moreover, a command started using this function can be interrupted via the Interrupt button in the interface which is not the case if the python
os.system()
function is used directly.If the command is given as one argument, it is converted to a string and passed to the system. If there are several arguments, each argument is converted to a string, surrounded by simple quotes and all elements are joined, separated by spaces. The resulting command is passed to the system. The second method is recommended because the usage of quotes enables to pass arguments that contain spaces. The function returns the value returned by the system command.
optional keyword paramerers
- outputLevel: (int)
if >=0 and < userLevel, write stdout in log
- stdoutInContext: (bool)
if True, write stdout in the current context output
- ignoreReturnValue: (bool)
if True, ignore the command return value. Useful when you know the command will exit badly even if the work is done.
- stdout: (file object)
if specified, stdout will be written in this stream. It may be a StringIO object.
- stderr: (file object)
if specified, stderr will be written in this stream. It may be a StringIO object.
- nativeEnv: (bool or None)
if True, forces execution within the “native” system environment variables (for commands external to the brainvisa binary distribution). If False, force execution within the current brainvisa environment. If None (default), guess if the executed command path is external to the main brainvisa path.
- cwd: (str or None)
Current directory of the child process (by default or if None, it is inherited from the parent process i.e. BrainVISA).
- env: dict
Environment variables to be set. Contrarily to soma.subprocess.Popen, they do not completely replace the current environment variables, but only add / replace the given variables. If both env and nativeEnv keyword arguments are used, nativeEnv acts before env, thus native environment can be overriden by env.
Example
>>> arg1 = 'x' >>> arg2 = 'y z' >>> context.system('command ' + arg1 + ' ' + arg2) >>> context.system('command', arg1, arg2)
The first call generates the command command x y z which calls the commands with 3 parameters. The second call generates the command ‘command’ ‘x’ ‘y z’ which calls the command with two parameters.
- temporary(format, diskItemType=None, suffix=None, prefix=None)[source]¶
This method enables to create a temporary DiskItem. The argument format is the temporary data format. The optional argument type is the data type. It generates one or several unique filenames (according to the format) in the temporary directory of BrainVISA (it can be changed in BrainVISA configuration). No file is created by this function. The process has to create it. The temporary files are deleted automatically when the temporary diskitem returned by the function is no later used.
Example
>>> tmp = context.temporary('GIS image') >>> context.runProcess('threshold', self.input, tmp, self.threshold) >>> tmp2 = context.temporary('GIS image') >>> context.system('AimsMorphoMath', '-m', 'ero', '-i', tmp.fullPath(), '-o', tmp2.fullPath(), '-r', self.size) >>> del tmp
In this example, a temporary data in GIS format is created and it is used to store the output of the process threshold. Then a new temporary data is created to store the output of a command line. At the end, the variable tmp is deleted, so the temporary data is no more referenced and the corresponding files are deleted.
- warning(*messages)[source]¶
This method is used to print a warning message. This function adds some HTML tags to change the appearance of the message and calls the
write()
function.
- write(*messages, **kwargs)[source]¶
This method is used to print information messages during the process execution. All arguments are converted into strings and joined to form the message. This message may contain HTML tags for an improved display. The result vary according to the context. If the process is run via its graphical interface, the message is displayed in the process window. If the process is run via a script, the message is displayed in the terminal. The message can also be ignored if the process is called automatically by brainvisa or another process.
context.write("Computing threshold of <i>", self.input.name, "</i>..." )
- class brainvisa.processes.ProcessTree(name=None, id=None, icon=None, tooltip=None, editable=True, user=True, content=[])[source]¶
Bases:
soma.notification.EditableTree
Represents a hierarchy of processes. It is used to represent the processes of a toolbox or a set of personal bookmarks on processes.
The tree contains branches: categories or directories, and leaves: processes.
This object can be saved in a minf file (in userProcessTree.minf for user bookmarks).
- Parameters:
name (string) – name of the tree
id (string) – id of the process. if None, it is set to the name in lowercase.
icon (string) – filename of an icon that represents the process tree.
tooltip (string) – description associated to the process tree
editable (boolean) – if True, the tree can be modified after its creation.
user (boolean) – if True, this tree is a custom process tree created by the user (personal bookmarks on processes)
content (list) – initial content, list of children to add in the tree.
- class Branch(name=None, id=None, editable=True, icon=None, content=[])[source]¶
Bases:
soma.notification.EditableTree.Branch
A directory that contains processes and/or another branches. Enables to organise processes by category.
All parameters must have default values to be able to create new elements automatically
- class Leaf(id, name=None, editable=True, icon=None, userLevel=None, *args, **kwargs)[source]¶
Bases:
soma.notification.EditableTree.Leaf
A ProcessTree.Leaf represents a process.
- addDir(processesDir, category='', processesCache={}, toolbox='brainvisa')[source]¶
Adds the processes from a directory to the current tree. Subdirectories will become the branches of the tree and processes will become the leaves of the tree.
- Parameters:
processesDir (string) – directory where processes are recursively searched.
category (string) – category prefix for all processes found in this directory (useful for toolboxes : all processes category begins with toolbox’s name.
processesCache (dictionary) – a dictionary containing previously saved processes info stored by id. Processes that are in this cache are not reread.
- setEditable(bool)[source]¶
Makes the tree editable. All its children becomes modifiable and deletable.
- setVisible()[source]¶
Sets the tree as visible if it is a user tree or if it has at least one visible child. An empty user tree is visible because it can be a newly created user tree and the user may want to fill it later.
- class brainvisa.processes.ProcessTrees(name=None)[source]¶
Bases:
ObservableAttributes
,ObservableSortedDictionary
Model for the list of process trees in brainvisa. A process tree is an instance of the class
ProcessTree
. It is a dictionary which maps each tree with its id. It contains several process trees :default process tree : all processes in brainvisa/processes (that are not in a toolbox). Not modifiable by user.
toolboxes : processes grouped by theme. Not modifiable by user.
user process trees (personal bookmarks): lists created by the user and saved in a minf file.
A tree can be set as default. It becomes the current tree at Brainvisa start.
- name¶
Name of the object.
- userProcessTreeMinfFile¶
Path to the file which stores the process trees created by the user as bookmarks. Default filename is in brainvisa home directory and is called userProcessTrees.minf.
- selectedTree¶
ProcessTree
that is the current tree when Brainvisa starts.
- Parameters:
name (string) – Name of the object. Default is ‘Toolboxes’.
- add(processTree)[source]¶
Add an item in the dictionary. If this item’s id is already present in the dictionary as a key, add the item’s content in the corresponding key. recursive method
- load()[source]¶
- Loads process trees :
a tree containing all processes that are not in toolboxes: the function
allProcessesTree()
returns it;toolboxes as new trees;
user trees that are saved in a minf file in user’s .brainvisa directory.
- Functions:
- brainvisa.processes.getProcessInfo(processId)[source]¶
Gets information about the process whose id is given in parameter.
- Return type:
- brainvisa.processes.allProcessesInfo()[source]¶
Returns a list of
ProcessInfo
objects for the loaded processes.
- brainvisa.processes.getProcess(processId, ignoreValidation=False, checkUpdate=True)[source]¶
Gets the class associated to the process id given in parameter.
When the processes are loaded, a new class called
NewProcess
is created for each process. This class inherits fromProcess
and adds an instance counter which is incremented each time a new instance of the process is created.- Parameters:
processId – the id or the name of the process, or a dictionary {‘type’ : ‘iteration|distributed|selection’, ‘children’ : […] } to create an
IterationProcess
, aDistributedProcess
or aSelectionProcess
.ignoreValidation (boolean) – if True the validation function of the process won’t be executed if the process need to be reloaded - default False.
checkUpdate (boolean) – If True, the modification date of the source file of the process will be checked. If the file has been modified since the process loading, it may need to be reloaded. The user will be asked what he wants to do. Default True.
- Returns:
a
NewProcess
class which inherits fromProcess
.
- brainvisa.processes.getProcessInstance(processIdClassOrInstance, ignoreValidation=False)[source]¶
Gets an instance of the process given in parameter.
- Parameters:
processIdClassOrInstance (a process id, name, class, instance, execution node, or a the name of a file containing a backup copy of a process.) –
ignoreValidation (bool (optional)) – if True, a validation failure will not prevent from building an instance of the process.
- Return type:
an instance of the
NewProcess
class associated to the described process.
- brainvisa.processes.getProcessInstanceFromProcessEvent(event)[source]¶
Gets an instance of a process described in a
brainvisa.history.ProcessExecutionEvent
.- Parameters:
event – a
brainvisa.history.ProcessExecutionEvent
that describes the process: its structure and its parameters.- Returns:
an instance of the
NewProcess
class associated to the described process. Parameters may have been set.
- brainvisa.processes.getProcessFromExecutionNode(node)[source]¶
Gets a process instance corresponding to the given execution node.
- Parameters:
node – a process
ExecutionNode
- Returns:
According to the type of node, it returns:
a
NewProcess
instance if the node isProcessExecutionNode
,an
IterationProcess
if the node is aSerialExecutionNode
a
DistributedProcess
if the node is aParallelExecutionNode
a
SelectionProcess
if the node is aSelectionExecutionNode
.
- brainvisa.processes.getConverter(source, destination, checkUpdate=True)[source]¶
Gets a converter (a process that have the role converter) which can convert data from source format to destination format. Such converters can be used to extend the set of formats that a process accepts.
- Parameters:
source – tuple (type, format). If a converter is not found directly, parent types are tried.
destination – tuple (type, format)
checkUpdate (boolean) – if True, Brainvisa will check if the converter needs to be reloaded. Default True.
- Returns:
the
NewProcess
class associated to the found converter.
- brainvisa.processes.getConvertersTo(dest, keepType=1, checkUpdate=True)[source]¶
Gets the converters which can convert data to destination format.
- Parameters:
destination – tuple (type, format). If a converter is not found directly, parent types are tried.
keepType (boolean) – if True, parent type won’t be tried. Default True.
checkUpdate (boolean) – if True, Brainvisa will check if the converters needs to be reloaded. Default True.
- Returns:
a dict (type, format) ->
NewProcess
class associated to the found converter.
- brainvisa.processes.getConvertersFrom(source, keepType=1, checkUpdate=True)[source]¶
Gets the converters which can convert data from source format to whatever format.
- Parameters:
source – tuple (type, format). If a converter is not found directly, parent types are tried.
checkUpdate (boolean) – if True, Brainvisa will check if the converters needs to be reloaded. Default True.
- Returns:
a dict (type, format) ->
NewProcess
class associated to the found converter.
- brainvisa.processes.getViewer(source, enableConversion=1, checkUpdate=True, listof=False, index=0, process=None, check_values=False)[source]¶
Gets a viewer (a process that have the role viewer) which can visualize source data. The viewer is returned only if its userLevel is lower than the current userLevel.
- Parameters:
source – a
neuroDiskItems.DiskItem
, a list ofneuroDiskItems.DiskItem
(only the first will be taken into account), a tuple (type, format).enableConversion (boolean) – if True, a viewer that accepts a format in which source can be converted is also accepted. Default True
checkUpdate (boolean) – if True, Brainvisa will check if the viewer needs to be reloaded. Default True.
listof (boolean) – If True, we need a viewer for a list of data. If there is no specific viewer for a list of this type of data, a
ListOfIterationProcess
is created from the associated simple viewer. Default False.index (int) – index of the viewer to find. Default 0.
process (None or NewProcess class or instance) – if specified, specialized viewers having a variable ‘allowed_processes’ which list this process, will be sorted first
check_values (bool) – if True, check if the 1st parameter of viewer actually accepts the source value. This is not always true because some filtering may happen using some requiredAttribues.
- Returns:
the
NewProcess
class associated to the found viewer or None if not found at the specified index.- Return type:
viewer
- brainvisa.processes.runViewer(source, context=None, process=None)[source]¶
Searches for a viewer for source data and runs the process. If viewer fail to display source, tries to get another.
- Parameters:
source – a
neuroDiskItems.DiskItem
or something that enables to find aneuroDiskItems.DiskItem
.context – the
ExecutionContext
. If None, the default context is used.
- Returns:
the result of the execution of the found viewer.
- brainvisa.processes.getDataEditor(source, enableConversion=0, checkUpdate=True, listof=False, index=0, process=None, check_values=False)[source]¶
Gets a data editor (a process that have the role editor) which can open source data for edition (modification). The data editor is returned only if its userLevel is lower than the current userLevel.
- Parameters:
source – a
neuroDiskItems.DiskItem
, a list ofneuroDiskItems.DiskItem
(only the first will be taken into account), a tuple (type, format).enableConversion (boolean) – if True, a data editor that accepts a format in which source can be converted is also accepted. Default False
checkUpdate (boolean) – if True, Brainvisa will check if the editor needs to be reloaded. Default True.
listof (boolean) – If True, we need an editor for a list of data. If there is no specific editor for a list of this type of data, a
ListOfIterationProcess
is created from the associated simple editor. Default False.process (None or NewProcess class or instance) – if specified, specialized viewers having a variable ‘allowed_processes’ which list this process, will be sorted first
check_values (bool) – if True, check if the 1st parameter of each data editor actually accepts the source value. This is not always true because some filtering may happen using some requiredAttribues.
- Returns:
editor
- Return type:
the
NewProcess
class associated to the found editor.
- brainvisa.processes.getImporter(source, checkUpdate=True)[source]¶
Gets a importer (a process that have the role importer) which can import data in the database.
- Parameters:
source – a
neuroDiskItems.DiskItem
or a tuple (type, format).checkUpdate (boolean) – if True, Brainvisa will check if the process needs to be reloaded. Default True.
- Returns:
the
NewProcess
class associated to the found process.
- brainvisa.processes.addProcessInfo(processId, processInfo)[source]¶
Stores information about the process.
- brainvisa.processes.readProcess(fileName, category=None, ignoreValidation=False, toolbox='brainvisa')[source]¶
Loads a process from its source file. The source file is a python file which defines some variables (signature, name, userLevel) and functions (validation, initialization, execution).
The process is indexed in the global lists of processes so it can be retrieved through the functions
getProcess()
,getProcessInfo()
,getViewer()
, …- Parameters:
fileName (string) – the name of the file containing the source code of the process.
category (string) – category of the process. If None, it is the name of the directory containing the process.
ignoreValidation (boolean) – if True, the validation function of the process won’t be executed.
toolbox (string) – The id of the toolbox containing the process. Defaut is ‘brainvisa’, it indicates that the process is not in a toolbox.
- Returns:
A
NewProcess
class representing the process if no exception is raised during the loading of the process.
A new class derived from
Process
is defined to store the content of the file:- class brainvisa.processes.NewProcess¶
Bases:
Process
All the elements defined in the file are added to the class.
- name¶
Name of the process. If it is not defined in the process file, it is the base name of the file without extension.
- category¶
The category of the process. If it is not given in parameter, it is the name of the directory containing the process file.
- dataDirectory¶
The data directory of the process is a directory near the process file with the same name and the extension .data. It is optional.
- processReloadNotifier¶
A
soma.notification.Notifier
that will notify its observers when the process is reload.
- signature¶
The parameters excepted by the process.
- userLevel¶
Minimum userLevel needed to see the process.
- roles¶
Roles of the process: viewer, converter, editor, impoter.
- execution(self, context)¶
Execution function.
- brainvisa.processes.readProcesses(processesPath)[source]¶
Read all the processes found in toolboxes and in a list of directories. The toolboxes are found with the function
brainvisa.toolboxes.allToolboxes()
.A global object representing a tree of processes is created, it is an instance of the
ProcessTree
- Parameters:
processesPath (list) – list of paths to directories containing processes files.
- brainvisa.processes.updatedMainProcessTree()[source]¶
- Return type:
- Returns:
Brainvisa list of process trees : all processes tree, toolboxes, user trees
- brainvisa.processes.allProcessesTree()[source]¶
Get the tree that contains all processes. It is created when processes in processesPath are first read. Toolboxes processes are also added in this tree.
- Return type:
- Returns:
the tree that contains all processes.
- brainvisa.processes.updateProcesses()[source]¶
Called when option userLevel has changed. Associated widgets will be updated automatically because they listens for changes.
- brainvisa.processes.defaultContext()[source]¶
Gets the default execution context.
- Return type:
- Returns:
The default execution context associated to Brainvisa application.
- brainvisa.processes.initializeProcesses()[source]¶
Intializes the global variables of the module. The current thread is stored as the main thread. A default execution context is created.
- brainvisa.processes.cleanupProcesses()[source]¶
Callback associated to the application exit. The global variables are cleaned.
- class brainvisa.processes.DiskItemConversionInfo(source, dest, checkUpdate=True)[source]¶
Bases:
object
Contains information about
neuroDiskItems.DiskItem
conversions. The conversion needs a source and a destinationThe object can be used to determine, if the conversion is possible, if it uses inheritance mechanisms, if it needs type conversion, if it needs format conversion.
If the conversion is possible, a distance between
neuroDiskItems.DiskItem
can be processed. This is useful to relevantly sort processes.DiskItemConversionInfo.- Parameters:
source – the source of
neuroDiskItems.DiskItem
conversion. It can be
neuroDiskItems.DiskItem
, a list ofneuroDiskItems.DiskItem
(only the first will be taken into account), a tuple (type, format). :param dest: the destination ofneuroDiskItems.DiskItem
conversion. It can beneuroDiskItems.DiskItem
, a list ofneuroDiskItems.DiskItem
(only the first will be taken into account), a tuple (type, format). :param boolean checkUpdate: if the convertersNewProcess
must be reloaded when they changed. Default True.- converter(exactConversionTypeOnly=False)[source]¶
Get converter between source and destination type if needed and possible.
- Returns:
the converter
NewProcess
if needed and possible
else None.
- distance(useInheritanceOnly=False, exactConversionTypeOnly=False)[source]¶
Gets a distance between source
neuroDiskItems.DiskItem
and destinationneuroDiskItems.DiskItem
of the processes.DiskItemConversionInfo. This is useful to relevantly sort processes.DiskItemConversionInfo- Parameters:
useInheritanceOnly (boolean) – Specify if the distance must be
processed only when
neuroDiskItems.DiskItemType
of the source inherits fromneuroDiskItems.DiskItemType
of the destination and does not need aNewProcess
converter call. Default False. :param boolean exactConversionTypeOnly: Specify if the conversion is valid only when converter is registered for the exact destinationneuroDiskItems.DiskItemType
. Default False- Returns:
None when conversion is not possible, else a tuple that
contains: 1) if the conversion needs a call to a
NewProcess
converter. When no converter call is needed, the value is 0, else the value is 1. 2) the distance between sourceneuroDiskItems.DiskItemType
and destinationneuroDiskItems.DiskItemType
. When source type is equal to destination type, the value is 0. When source inherits from destination and no conversion is needed, the value is the number of levels between them, else the value is 1. 3) if the sourceneuroDiskItems.Format
is identical to destinationneuroDiskItems.Format
value is 0, else 1
- exactDestTypeConverter()[source]¶
Get converter between source and exact destination type if needed and possible.
- Returns:
the converter
NewProcess
to exact destination
type if needed and possible else None.
- exists(useInheritanceOnly=False, exactConversionTypeOnly=False)[source]¶
Checks that a conversion between source
neuroDiskItems.DiskItem
and destinationneuroDiskItems.DiskItem
exists.- Parameters:
useInheritanceOnly (boolean) – Specify if the check must only
consider inheritance between source
neuroDiskItems.DiskItemType
and destinationneuroDiskItems.DiskItemType
, without checking existing converterNewProcess
. Default False. :param boolean exactConversionTypeOnly: Specify if the conversion is valid only when converter is registered for the exact destinationneuroDiskItems.DiskItemType
. Default False- Returns:
True if conversion is possible between source
neuroDiskItems.DiskItem
and destinationneuroDiskItems.DiskItem
, else False
- class brainvisa.processes.DistributedProcess(name, processes)[source]¶
Bases:
Process
This class represents a set of process instances that can be executed in parallel.
- class brainvisa.processes.ExecutionContext(userLevel=None, debug=None)[source]¶
Bases:
object
This object represents the execution context of the processes.
Indeed, a process can be started in different contexts :
The user starts the process by clicking on the Run button in the graphical interface.
The process is started via a script. It is possible to run brainvisa in batch mode (without any graphical interface) and to run a process via a python function : brainvisa.processes.defaultContext().runProcess(…).
The process is a converter, so it can be run automatically by BrainVISA when a conversion is needed for another process parameters.
The process is a viewer or an editor, it is run when the user clicks on the corresponding icon to view or edit another process parameter.
The interactions with the user are different according to the context. That’s why the context object offers several useful functions to interact with BrainVISA and to call system commands. Here are these functions :
write()
,warning()
,error()
: prints a message, either in the graphical process window (in GUI mode) or in the terminal (in batch mode).log()
: writes a message in the BrainVISA log file.temporary()
: creates a temporary file.system()
: calls a system command.runProcess()
: runs a BrainVISA process.checkInterruption()
: defines a breakpoint.
- ask(message, *buttons, **kwargs)[source]¶
This method asks a question to the user. The message is displayed and the user is invited to choose a value among the propositions. The method returns the index of the chosen value, beginning by 0. If the answer is not valid, the returned value is -1. Sometimes, when the process is called automatically (in batch mode), these calls to context.ask are ignored and return directly -1 without asking question.
Example
>>> if context.ask('Is the result ok ?', 'yes', 'no') == 1: >>> try_again()
- checkInterruption()[source]¶
This function is used to define breakpoints. When the process execution reach a breakpoint, the user can interrupt the process. There are 4 types of breakpoints automatically added :
before each system call (context.system)
after each system call (context.system)
before each sub-process call (context.runProcess)
after each sub-process call (context.runProcess)
To allow the user to interrupt the process at another place, you have to use the function context.checkInterruption. If the user has clicked on the Interrupt button while the process runs, it will stop when reaching the checkInterruption point.
- dialog(*args)[source]¶
This method is available only in a graphical context. Like ask, it is used to ask a question to the user, but the dialog interface is customisable. It is possible to add a signature to the dialog : fields that the user has to fill in.
Example
>>> dial = context.dialog(1, 'Enter a value', Signature('param', Number()), _t_('OK'), _t_('Cancel')) >>> dial.setValue('param', 0) >>> r = dial.call() >>> if r == 0: >>> v=dial.getValue('param')
- error(*messages)[source]¶
This method is used to print an error message. Like the above function, it adds some HTML tags to change the appearance of the message and calls
write()
function.
- getConverter(source, dest, checkUpdate=True)[source]¶
Gets a converter process which can convert the source diskitem from its format to the destination format.
- getProgressInfo(process, childrencount=None, parent=None)[source]¶
Get the progress info for a given process or execution node, or create one if none already exists. A regular process may call it.
The output is a tuple containing the ProgressInfo and the process itself, just in case the input process is in fact a ProgressInfo instance. A ProgressInfo has no hard reference in BrainVISA: when you don’t need it anymore, it is destroyed via Python reference counting, and is considered done 100% for its parent.
- Parameters:
childrencount – it is the number of children that the process will have, and is not the same as the own count of the process in itself, which is in addition to children (and independent), and specified when using the progress() method.
- log(*args, **kwargs)[source]¶
context.log(what, when=None, html=’’, children=[], icon=None)
This method is used to add a message to BrainVISA log. The first parameter what is the name of the entry in the log, the message to write is in the html parameter.
- progress(value=None, count=None, process=None)[source]¶
Set the progress information for the parent process or ProgressInfo instance, and output it using the context output mechanisms.
- Parameters:
value – is the progress value to set. If none, the value will not be changed, but the current status will be shown.
count – is the maximum value for the process own progress value (not taking children into account).
process – is either the calling process, or the ProgressInfo.
- pythonSystem(*args, **kwargs)[source]¶
Specialization of system() for calling Python scripts
The script passed as the first agrument is called through the Python interpreter (
sys.executable
). The script will be searched inPATH
and its full path will be passed to the interpreter, unless the first argument begins with-
(which allows using-c
or-m
),On Unix, this should have the same result as using system(), but on Windows the script may not be recognized as a Python script by the system, so needs this wrapping.
NOTE: this method will always use the same interpreter as BrainVISA (i.e.
sys.executable
), regardless of the shebang line in your Python script. Therefore, you should make sure that your script is compatible with both Python 2 and Python 3.
- runInteractiveProcess(callMeAtTheEnd, process, *args, **kwargs)[source]¶
Runs a process in a new thread and calls a callback function when the execution is finished.
- Parameters:
callMeAtTheEnd (function) – callback function which will be called the process execution is finished.
process – id of the process which will be run.
- runProcess(_process, *args, **kwargs)[source]¶
It is possible to call a sub-process in the current process by calling context.runProcess.
The first argument is the process identifier, which is either the filename wihtout extension of the process or its english name. The other arguments are the values of the process parameters. All mandatory argument must have a value. The function returns the value returned by the sub-process execution method.
Example
>>> context.runProcess('do_something', self.input, self.output, value=3.14)
In this example, the process do_something is called with self.input as the first paramter value, self.ouput as the second parameter value and 3.14 to the parameter named value.
- showException(beforeError='', afterError='', exceptionInfo=None)[source]¶
same as the global brainvisa.processing.neuroException.showException() but displays in the current context (the process output box for instance)
- showProgress(value, count=None)[source]¶
Output the given progress value. This is just the output method which is overriden in subclassed contexts.
Users should normally not call it directory, but use progress() instead.
- system(*args, **kwargs)[source]¶
This function is used to call system commands. It is very similar to functions like
os.system()
in Python andsystem()
in C. The main difference is the management of messages sent on standard output. These messages are intercepted and reported in BrainVISA interface according to the current execution context.Moreover, a command started using this function can be interrupted via the Interrupt button in the interface which is not the case if the python
os.system()
function is used directly.If the command is given as one argument, it is converted to a string and passed to the system. If there are several arguments, each argument is converted to a string, surrounded by simple quotes and all elements are joined, separated by spaces. The resulting command is passed to the system. The second method is recommended because the usage of quotes enables to pass arguments that contain spaces. The function returns the value returned by the system command.
optional keyword paramerers
- outputLevel: (int)
if >=0 and < userLevel, write stdout in log
- stdoutInContext: (bool)
if True, write stdout in the current context output
- ignoreReturnValue: (bool)
if True, ignore the command return value. Useful when you know the command will exit badly even if the work is done.
- stdout: (file object)
if specified, stdout will be written in this stream. It may be a StringIO object.
- stderr: (file object)
if specified, stderr will be written in this stream. It may be a StringIO object.
- nativeEnv: (bool or None)
if True, forces execution within the “native” system environment variables (for commands external to the brainvisa binary distribution). If False, force execution within the current brainvisa environment. If None (default), guess if the executed command path is external to the main brainvisa path.
- cwd: (str or None)
Current directory of the child process (by default or if None, it is inherited from the parent process i.e. BrainVISA).
- env: dict
Environment variables to be set. Contrarily to soma.subprocess.Popen, they do not completely replace the current environment variables, but only add / replace the given variables. If both env and nativeEnv keyword arguments are used, nativeEnv acts before env, thus native environment can be overriden by env.
Example
>>> arg1 = 'x' >>> arg2 = 'y z' >>> context.system('command ' + arg1 + ' ' + arg2) >>> context.system('command', arg1, arg2)
The first call generates the command command x y z which calls the commands with 3 parameters. The second call generates the command ‘command’ ‘x’ ‘y z’ which calls the command with two parameters.
- temporary(format, diskItemType=None, suffix=None, prefix=None)[source]¶
This method enables to create a temporary DiskItem. The argument format is the temporary data format. The optional argument type is the data type. It generates one or several unique filenames (according to the format) in the temporary directory of BrainVISA (it can be changed in BrainVISA configuration). No file is created by this function. The process has to create it. The temporary files are deleted automatically when the temporary diskitem returned by the function is no later used.
Example
>>> tmp = context.temporary('GIS image') >>> context.runProcess('threshold', self.input, tmp, self.threshold) >>> tmp2 = context.temporary('GIS image') >>> context.system('AimsMorphoMath', '-m', 'ero', '-i', tmp.fullPath(), '-o', tmp2.fullPath(), '-r', self.size) >>> del tmp
In this example, a temporary data in GIS format is created and it is used to store the output of the process threshold. Then a new temporary data is created to store the output of a command line. At the end, the variable tmp is deleted, so the temporary data is no more referenced and the corresponding files are deleted.
- warning(*messages)[source]¶
This method is used to print a warning message. This function adds some HTML tags to change the appearance of the message and calls the
write()
function.
- write(*messages, **kwargs)[source]¶
This method is used to print information messages during the process execution. All arguments are converted into strings and joined to form the message. This message may contain HTML tags for an improved display. The result vary according to the context. If the process is run via its graphical interface, the message is displayed in the process window. If the process is run via a script, the message is displayed in the terminal. The message can also be ignored if the process is called automatically by brainvisa or another process.
context.write("Computing threshold of <i>", self.input.name, "</i>..." )
- class brainvisa.processes.ExecutionNode(name='', optional=False, selected=True, guiOnly=False, parameterized=None, expandedInGui=False)[source]¶
Bases:
object
Base class for the classes that describe a pipeline structure.
- Parameters:
name (str) – name of the node - default ‘’.
optional (boolean) – indicates if this node is optional in the pipeline - default False.
selected (boolean) – indicates if the node is selected in the pipeline - default True.
guiOnly (boolean) – default False.
parameterized –
Parameterized
containing the signature of the node - default None.
- addChild(name, node, index=None)[source]¶
Add a new child execution node.
- Parameters:
name (string) – name which identifies the node
node – an
ExecutionNode
which will be added to this node’s children.
- addDoubleLink(destination, source, function=None)[source]¶
Creates a double link source -> destination and destination -> source.
- addExecutionDependencies(deps)[source]¶
Adds to the execution node dependencies on the execution of other nodes. This allows to build a dependencies structure which is not forced to be a tree, but can be a grapĥ. Dependencies are used to build Soma-Workflow workflows with correct dependencies.
- addLink(destination, source, function=None, destDefaultUpdate=True)[source]¶
Adds a parameter link like
Parameterized.addLink()
.
- allParameterFiles()[source]¶
Get recursively all parameters which are DiskItems, descending through the pipeline structure.
- property parameterized¶
The Parameterized object associated with this node.
The ExecutionNode keeps only a weak reference in _parameterized. This property returns a strong reference to the Parameterized object if it is still alive, otherwise it returns None.
- parent_pipeline()[source]¶
Returns the root pipeline process from which this node is part of, if it is actually part of a pipeline (otherwise None is returned).
New in Axon 4.6.
- parseParameterString(parameterString)[source]¶
Returns a tuple containing the
Parameterized
object of the child node indicated in the parameter string and the name of the parameter. May return None if the node is invalid.- Parameters:
parameterString (str) – references a parameter of a child node with a path like <node name 1>.<node name 2>…<parameter name>
- removeChild(name)[source]¶
Remove child execution node.
- Parameters:
name (string) – name which identifies the node
- removeDoubleLink(destination, source, function=None, show_warnings=True)[source]¶
Removes a double link source -> destination and destination -> source.
- class brainvisa.processes.IterationExecutionNode(base, name=None, hidechildren=False, optional=False, selected=True, notify=True)[source]¶
Bases:
ParallelExecutionNode
- Parameters:
name (str) – name of the node - default ‘’.
optional (boolean) – indicates if this node is optional in the pipeline - default False.
selected (boolean) – indicates if the node is selected in the pipeline - default True.
guiOnly (boolean) – default False.
parameterized –
Parameterized
containing the signature of the node - default None.
- class IterationParameterized(base)[source]¶
Bases:
Parameterized
- class brainvisa.processes.IterationProcess(name, processes, base=None)[source]¶
Bases:
Process
This class represents a set of process instances that can be executed in parallel.
It is used to iterate the same process on a set of data.
- class brainvisa.processes.ListOfIterationProcess(name, processes)[source]¶
Bases:
IterationProcess
An IterationProcess which has on its main signature a list of the first element of each sub-process.
Used for viewers and editors of ListOf()
- class brainvisa.processes.ParallelExecutionNode(name='', optional=False, selected=True, guiOnly=False, parameterized=None, stopOnError=True, expandedInGui=False, possibleChildrenProcesses=None, notify=False)[source]¶
Bases:
SerialExecutionNode
An execution node that run all its children in any order (and in parallel if possible)
- Parameters:
name (str) – name of the node - default ‘’.
optional (boolean) – indicates if this node is optional in the pipeline - default False.
selected (boolean) – indicates if the node is selected in the pipeline - default True.
guiOnly (boolean) – default False.
parameterized –
Parameterized
containing the signature of the node - default None.
- class brainvisa.processes.Parameterized(signature)[source]¶
Bases:
object
This class represents an object that have a signature, that is to say a list of typed parameters.
A Parameterized object can notify the changes in its signature. The parameters can be linked together, that is to say, if the value of a parameter changes, the value of all linked parameters may change.
This object has an
initialization()
function that can define the links between the parameters and their initial values.- Attributes:
- signature¶
The signature is a
brainvisa.data.neuroData.Signature
. It contains the list of parameters accepted by the object and their types. The possible types are described inbrainvisa.data.neuroData
.
- signatureChangeNotifier¶
This variable is a
soma.notification.Notifier
. It calls its notify function when the signature of theParameterized
object changes.
- addDoubleLink(destination, source, function=None)[source]¶
Creates a double link source -> destination and destination -> source.
- addLink(destination, source, function=None, destDefaultUpdate=True)[source]¶
Add a link between source and destination parameters. When the value of source changes, the value of destination may change. Contrary to
linkParameters()
, the link will always be applied, even if the destination parameter has no more its default value.- Parameters:
destination (str) – name of the parameter that may change when the source parameters change. If None, the link function will be called every time the source parameters change.
source (str, tuple or list) – one or several parameters, whose modification will activate the link function.
function (function) – specific function that will be called instead of the default one when the link is activated. The signature of the function is function(self, *sources ) -> destination
destDefaultUpdate (bool) – specify that destination attribute will be marked as manually changed if the default value was changed by the link.
- addParameterObserver(parameterName, function)[source]¶
Associates a callback function to the modifications of the parameter value.
- Parameters:
parameterName (str) – the name of the parameter whose modification will activate the callback.
function (function) – the callback function. its signature is function(self, parameterName, newValue)
- blockLinks(blocked)[source]¶
While links are blocked, calls to setValue() or other parameters changes do not trigger links.
- changeSignature(signature)[source]¶
Sets a new signature. Previous values of attributes are kept if the attributes are still in the signature. Links and observer callbacks that are no more associated to the signature parameters are deleted. The
signatureChangeNotifier
is notified.
- cleanup()[source]¶
Removes all links, observers, and stored converted values, reinitializes the signature change notifier.
- findValue(attributeName, value)[source]¶
Calls
setValue()
.
- initialization()[source]¶
This function does nothing by default but it may be overrideed in processes classes to define initial values for the parameters or links between parameters.
- linkParameters(destName, sources, function=None, link_attributes={})[source]¶
Links the parameters. When one of the sources parameters change, the value of destName parameter may change. It is possible to give a specific link function that will be called when the link is applied but it is not mandatory, a default function exists according to the type of parameter.
- Parameters:
destName (string) – name of the parameter that may change when the sources parameters change. If None, the link function will be called every time the source parameters change.
sources (string, tuple or list of strings) – one or several parameters, whose modification will activate the link function.
function (function) – specific function to call instead of the default one when the link is activated. The signature of the function is function(self, process), returning destination
link_attributes (dict (optional)) –
A dictionary of mandatory linked attributes. This is only meaningful for DiskItem parameters, which have attributes. Attributes listed here will be added as requiredAttributes to ReadDiskItem.findValue(). The dict maps destination attributes from source parameters attributes values. Ex: * {‘dst_attrib’: ‘src_attrib’}
will get in the source parameter value the attribute ‘src_attrib’, and its value will be forced as the value of the ‘dst_attrib’ attribute of the destination parameter.
- {‘dst_attrib1’: ‘src_param1:src_attrib1’,
’dst_attrib2’: ‘src_param2:src_attrib2’}
will get the attribute value of ‘src_attrib1’ in source parameter ‘src_param1’, and set it as the value of ‘dst_attrib1’ of the destination parameter. Same for ‘dst_attrib2’, but the value will be taken from another source parameter.
As values are passed as requiredAttributes, they are thus mandatory in the destination parameter value, and are “stronger” than standard links. In this respect, it can be meaningful to get an attribute which has already the same name/value in the source parameter: {‘my_attrib’: ‘my_attrib’} will just reject values with non-matching attribute ‘my_attrib’ (compared to a standard link).
- parameterLinkable(key, debug=None)[source]¶
Indicates if the value of the parameter can change through a parameter link.
- removeDoubleLink(destination, source, show_warnings=True)[source]¶
Removes a double link source -> destination and destination -> source.
- removeLink(destination, source, show_warnings=True)[source]¶
Removes a link added with
addLink()
function.
- removeParameterObserver(parameterName, function)[source]¶
Removes the callback function from the parameter observers.
- restoreConvertedValues()[source]¶
Restore values as they were before conversions using the values stored in an internal dictionary.
- setConvertedValue(name, value)[source]¶
Sets the value but stores the previous value in an internal dictionary.
- setEnable(*args, **kwargs)[source]¶
Indicates parameters visibility and mandatory using examples : self.setEnable( *args)
optional keyword paramerers
- userLevel: int
indicates that the parameters are visible or hidden regarding the userLevel. ( default value : the previous userLevel is kept )
- mandatory: boolean
indicates that the parameters are mandatory(True) or optional(False). ( default value : True )
- setSection(section, *args)[source]¶
Sets the section of the parameters. Parameters are then sorted by section in the GUI
- class brainvisa.processes.Process[source]¶
Bases:
Parameterized
This class represents a Brainvisa process or pipeline.
This object has a signature that describes its inputs and outputs and an execution function
execution()
. If it is a pipeline, it also have an execution node that describes the structure of the pipeline.- Attributes:
- signature¶
The signature is a
brainvisa.data.neuroData.Signature
. It contains the list of parameters accepted by the object and their types. The possible types are described inbrainvisa.data.neuroData
.
- category(string)¶
The processes are organized into categories. Generally, the category is the name of the directory where the process file is located.
- userLevel(integer)¶
The process is available in Brainvisa interface if its userLevel is lower or equal than the userLevel selected in Brainvisa options. 0 : Basic, 1: Advanced, 2: Expert.
- showMaximized(boolean)¶
If true, the process window is shown maximized with a frame around it.
- Methods:
- validation()[source]¶
This method can be overrideed in order to check if the process dependencies are available. It will be called at Brainvisa startup when the processes are loaded. If the method raises an exception, the process will not be available.
- setExecutionNode(eNode)[source]¶
Sets the execution node of the pipeline.
- Parameters:
eNode (
ExecutionNode
) – object that describes the structure of the pipeline.
- getAllParameters()[source]¶
Returns all the parameters of the current process and its children if it is a pipeline.
- Returns:
tuples (Parameterized, attribute name, attribute type)
- Return type:
generator
- allProcesses()[source]¶
Returns the current process and all its children if it is a pipeline.
- Return type:
generator
- pipelineStructure()[source]¶
Returns the description of a pipeline in a dictionary or the id of the process if it is a simple process.
- inlineGUI(values, context, parent, externalRunButton=False)[source]¶
This method can be overrideed in order to specialize buttons of the process window.
- Parameters:
context – the execution context of the process
parent – The parent widget
- Returns:
widget – the widget containing the buttons that will replace the default buttons (Run and Iterate)
- Return type:
QWidget
- _iterate(warn=True, **kwargs)[source]¶
Returns a list of copies of the current process with different parameters values.
- Parameters:
warn (bool) – raise an exception if iterated parameters lists sizes do not match
kwargs – dictionary containing a list of values for each parameter name. The first value is for the first process of the iteration and so on…
- _copy(withparams=True)[source]¶
Returns a copy of the process. The value of the parameters are also copied if withparams is True (which is the default)
- addLink(destination, source, function=None, destDefaultUpdate=True)[source]¶
Add a link between source and destination parameters. When the value of source changes, the value of destination may change. Contrary to
linkParameters()
, the link will always be applied, even if the destination parameter has no more its default value.- Parameters:
destination (str) – name of the parameter that may change when the source parameters change. If None, the link function will be called every time the source parameters change.
source (str, tuple or list) – one or several parameters, whose modification will activate the link function.
function (function) – specific function that will be called instead of the default one when the link is activated. The signature of the function is function(self, *sources ) -> destination
destDefaultUpdate (bool) – specify that destination attribute will be marked as manually changed if the default value was changed by the link.
- allParameterFiles()[source]¶
Get recursively all parameters which are DiskItems, descending through the pipeline structure.
- allProcesses()[source]¶
Returns the current process and all its children if it is a pipeline.
- Return type:
generator
- getAllParameters()[source]¶
Returns all the parameters of the current process and its children if it is a pipeline.
- Returns:
tuples (Parameterized, attribute name, attribute type)
- Return type:
generator
- inlineGUI(values, context, parent, externalRunButton=False)[source]¶
This method can be overrideed in order to specialize buttons of the process window.
- Parameters:
context – the execution context of the process
parent – The parent widget
- Returns:
widget – the widget containing the buttons that will replace the default buttons (Run and Iterate)
- Return type:
QWidget
- parent_pipeline()[source]¶
Returns the root pipeline process from which this process is part of, if it is actually part of a pipeline (otherwise None is returned).
New in Axon 4.6.
- pipelineStructure()[source]¶
Returns the description of a pipeline in a dictionary or the id of the process if it is a simple process.
- setExecutionNode(eNode)[source]¶
Sets the execution node of the pipeline.
- Parameters:
eNode (
ExecutionNode
) – object that describes the structure of the pipeline.
- class brainvisa.processes.ProcessExecutionNode(process, optional=False, selected=True, guiOnly=False, expandedInGui=False, altname=None, skip_invalid=False)[source]¶
Bases:
ExecutionNode
An execution node that has no children and run one process
- Parameters:
process (process id or instance or class) –
optional (bool) – may be unchecked in the pipeline (not run)
selected (bool) – checked in the pipeline, will be the selected one in a selection node
guiOnly (bool) – will be skipped in a non-interactive environment
expandedInGui (bool) – in the tree widget
altname (str) – alternative name displayed in the GUI
skip_invalid (bool) – marks the node as “optional”: if the process cannot be instantiated, the pipeline construction will not fail, but the node will not be added. Links involving this node will be also skipped silently. This is useful to buils pipelines with several alternative nodes which may be unavailable in some contexts (due to missing dependencies or external software, typically)
- addChild(name, node, index=None)[source]¶
Add a new child execution node.
- Parameters:
name (string) – name which identifies the node
node – an
ExecutionNode
which will be added to this node’s children.
- class brainvisa.processes.ProcessInfo(id, name, signature, userLevel, category, fileName, roles, toolbox, module=None, showMaximized=False)[source]¶
Bases:
object
This object stores information about a process. Such objects are created at BrainVISA startup when the processes are loaded.
- id¶
Id of the process. It is the name of the file without extension in lowercase.
- name¶
Name of the process as it is displayed in the GUI.
- signature¶
Process excepted parameters.
- userLevel¶
User level needed to see the process.
- category¶
Process category path: <toolbox>/<category1>/<category2>/…
- showMaximized¶
Process window maximized state.
- fileName¶
Path to the file containing the source code of the process.
- roles¶
Tuple containing the specific roles of the process: viewer, converter, editor, importer.
- valid¶
False if the validation method of the process fails - default True.
- procdoc¶
The content of the .procdoc file associated to this process in a dictionary. It represents the documentation of the process.
- toolbox¶
The id of the toolbox containing the process.
- module¶
Module path to the source of the process related to the toolbox directory. <processes>.<category1>…<process>
- class brainvisa.processes.ProcessSet(type, format, ids=(), listof=False)[source]¶
Bases:
set
Bases:
set
Set of processes that can process
neuroDiskItems.DiskItem
of a particularneuroDiskItems.DiskItemType
andneuroDiskItems.Format
:param
neuroDiskItems.DiskItemType
type: type ofneuroDiskItems.DiskItem
that can be used by the set of processes. :paramneuroDiskItems.Format
format: format ofneuroDiskItems.DiskItem
that can be used by the processes of the ProcessSet. :param tuple ids: identifiers of processesNewProcess
of the ProcessSet. :param bool listof: if the registered processes are able to process a list ofneuroDiskItems.DiskItem
or not.- accept(source, enableConversion=1, exactConversionTypeOnly=False, checkUpdate=True)[source]¶
Check that a
neuroDiskItems.DiskItem
source is processable using the processes of the current ProcessSet- Parameters:
enableConversion (int) – if the source can be converted to be
processed by the processes of the current ProcessSet. Default 1. :param boolean checkUpdate: if the converters
NewProcess
must be reloaded when they changed. Default True. :param boolean exactConversionTypeOnly: Specify if the conversion is valid only when converter is registered for the exact destinationneuroDiskItems.DiskItemType
. Default False- Returns:
True if the source is processable using the processes of the
current ProcessSet, else False.
- processes(checkUpdate=True)[source]¶
Get processes associated to the current ProcessSet and filtered for the current user level
- Parameters:
checkUpdate (boolean) – if the processes
NewProcess
must be reloaded when they changed. Default True.
- Returns:
a list of processes
NewProcess
associated to the
current ProcessSet and filtered for the current user level.
- class brainvisa.processes.ProcessTree(name=None, id=None, icon=None, tooltip=None, editable=True, user=True, content=[])[source]¶
Bases:
EditableTree
Bases:
soma.notification.EditableTree
Represents a hierarchy of processes. It is used to represent the processes of a toolbox or a set of personal bookmarks on processes.
The tree contains branches: categories or directories, and leaves: processes.
This object can be saved in a minf file (in userProcessTree.minf for user bookmarks).
- Parameters:
name (string) – name of the tree
id (string) – id of the process. if None, it is set to the name in lowercase.
icon (string) – filename of an icon that represents the process tree.
tooltip (string) – description associated to the process tree
editable (boolean) – if True, the tree can be modified after its creation.
user (boolean) – if True, this tree is a custom process tree created by the user (personal bookmarks on processes)
content (list) – initial content, list of children to add in the tree.
- class Branch(name=None, id=None, editable=True, icon=None, content=[])[source]¶
Bases:
Branch
Bases:
soma.notification.EditableTree.Branch
A directory that contains processes and/or another branches. Enables to organise processes by category.
All parameters must have default values to be able to create new elements automatically
- class Leaf(id, name=None, editable=True, icon=None, userLevel=None, *args, **kwargs)[source]¶
Bases:
Leaf
Bases:
soma.notification.EditableTree.Leaf
A ProcessTree.Leaf represents a process.
- addDir(processesDir, category='', processesCache={}, toolbox='brainvisa')[source]¶
Adds the processes from a directory to the current tree. Subdirectories will become the branches of the tree and processes will become the leaves of the tree.
- Parameters:
processesDir (string) – directory where processes are recursively searched.
category (string) – category prefix for all processes found in this directory (useful for toolboxes : all processes category begins with toolbox’s name.
processesCache (dictionary) – a dictionary containing previously saved processes info stored by id. Processes that are in this cache are not reread.
- setEditable(bool)[source]¶
Makes the tree editable. All its children becomes modifiable and deletable.
- setVisible()[source]¶
Sets the tree as visible if it is a user tree or if it has at least one visible child. An empty user tree is visible because it can be a newly created user tree and the user may want to fill it later.
- class brainvisa.processes.ProcessTrees(name=None)[source]¶
Bases:
ObservableAttributes
,ObservableSortedDictionary
Model for the list of process trees in brainvisa. A process tree is an instance of the class
ProcessTree
. It is a dictionary which maps each tree with its id. It contains several process trees :default process tree : all processes in brainvisa/processes (that are not in a toolbox). Not modifiable by user.
toolboxes : processes grouped by theme. Not modifiable by user.
user process trees (personal bookmarks): lists created by the user and saved in a minf file.
A tree can be set as default. It becomes the current tree at Brainvisa start.
- name¶
Name of the object.
- userProcessTreeMinfFile¶
Path to the file which stores the process trees created by the user as bookmarks. Default filename is in brainvisa home directory and is called userProcessTrees.minf.
- selectedTree¶
ProcessTree
that is the current tree when Brainvisa starts.
- Parameters:
name (string) – Name of the object. Default is ‘Toolboxes’.
- add(processTree)[source]¶
Add an item in the dictionary. If this item’s id is already present in the dictionary as a key, add the item’s content in the corresponding key. recursive method
- load()[source]¶
- Loads process trees :
a tree containing all processes that are not in toolboxes: the function
allProcessesTree()
returns it;toolboxes as new trees;
user trees that are saved in a minf file in user’s .brainvisa directory.
- class brainvisa.processes.ProgressInfo(parent=None, count=None, process=None)[source]¶
Bases:
object
ProgressInfo is a tree-like structure for progression information in a process or a pipeline. The final goal is to provide feedback to the user via a progress bar. ProgressInfo has children for sub-processes (when used in a pipeline), or a local value for its own progression.
A ProgressInfo normally registers itself in the calling Process, and is destroyed when the process is destroyed, or when the process _progressinfo variable is deleted.
- Parameters:
parent – is a ProgressInfo instance.
count – is a number of children which will be attached.
process – is the calling process.
- class brainvisa.processes.SelectionExecutionNode(*args, **kwargs)[source]¶
Bases:
ExecutionNode
An execution node that run one of its children
- Parameters:
name (str) – name of the node - default ‘’.
optional (boolean) – indicates if this node is optional in the pipeline - default False.
selected (boolean) – indicates if the node is selected in the pipeline - default True.
guiOnly (boolean) – default False.
parameterized –
Parameterized
containing the signature of the node - default None.
- class brainvisa.processes.SelectionProcess(name, processes)[source]¶
Bases:
Process
This class represents a choice between a list of processes.
- class brainvisa.processes.SerialExecutionNode(name='', optional=False, selected=True, guiOnly=False, parameterized=None, stopOnError=True, expandedInGui=False, possibleChildrenProcesses=None, notify=False)[source]¶
Bases:
ExecutionNode
An execution node that run all its children sequentially
- Parameters:
name (str) – name of the node - default ‘’.
optional (boolean) – indicates if this node is optional in the pipeline - default False.
selected (boolean) – indicates if the node is selected in the pipeline - default True.
guiOnly (boolean) – default False.
parameterized –
Parameterized
containing the signature of the node - default None.
- addChild(name=None, node=None, index=None)[source]¶
Add a new child execution node.
- Parameters:
name (string) – name which identifies the node
node – an
ExecutionNode
which will be added to this node’s children.
- brainvisa.processes.addProcessInfo(processId, processInfo)[source]¶
Stores information about the process.
- brainvisa.processes.allProcessesInfo()[source]¶
Returns a list of
ProcessInfo
objects for the loaded processes.
- brainvisa.processes.allProcessesTree()[source]¶
Get the tree that contains all processes. It is created when processes in processesPath are first read. Toolboxes processes are also added in this tree.
- Return type:
- Returns:
the tree that contains all processes.
- brainvisa.processes.cleanupProcesses()[source]¶
Callback associated to the application exit. The global variables are cleaned.
- brainvisa.processes.convertSpecialLinks(msg, language, baseForLinks, translator)[source]¶
Converts special links and tags in a procdoc documentation. The possible special links or tags are:
bvcategory:// refers to the documentation of a processes category (directory containing processes).
bvprocess:// refers to the documentation of a process
bvimage:// refers to an image in Brainvisa images directory
<_t_> translates the string in the selected language
*<bvprocessname name=””> replaces the id by the name of the process
- brainvisa.processes.defaultContext()[source]¶
Gets the default execution context.
- Return type:
- Returns:
The default execution context associated to Brainvisa application.
- brainvisa.processes.generateHTMLProcessesDocumentation(procId=None)[source]¶
Generates HTML pages for the documentation of the process in parameter or for all processes if procId is None. The process generateDocumentation is used.
- brainvisa.processes.getConversionInfo(source, dest, checkUpdate=True)[source]¶
Gets information about conversion of a source
neuroDiskItems.DiskItem
and destinationneuroDiskItems.DiskItem
.- Parameters:
source – the source of
neuroDiskItems.DiskItem
conversion. It can be
neuroDiskItems.DiskItem
, a list ofneuroDiskItems.DiskItem
(only the first will be taken into account), a tuple (type, format). :param dest: the destination ofneuroDiskItems.DiskItem
conversion. It can beneuroDiskItems.DiskItem
, a list ofneuroDiskItems.DiskItem
(only the first will be taken into account), a tuple (type, format). :param boolean checkUpdate: if the convertersNewProcess
must be reloaded when they changed. Default True.- Returns:
a
processes.DiskItemConversionInfo
- brainvisa.processes.getConverter(source, destination, checkUpdate=True)[source]¶
Gets a converter (a process that have the role converter) which can convert data from source format to destination format. Such converters can be used to extend the set of formats that a process accepts.
- Parameters:
source – tuple (type, format). If a converter is not found directly, parent types are tried.
destination – tuple (type, format)
checkUpdate (boolean) – if True, Brainvisa will check if the converter needs to be reloaded. Default True.
- Returns:
the
NewProcess
class associated to the found converter.
- brainvisa.processes.getConvertersFrom(source, keepType=1, checkUpdate=True)[source]¶
Gets the converters which can convert data from source format to whatever format.
- Parameters:
source – tuple (type, format). If a converter is not found directly, parent types are tried.
checkUpdate (boolean) – if True, Brainvisa will check if the converters needs to be reloaded. Default True.
- Returns:
a dict (type, format) ->
NewProcess
class associated to the found converter.
- brainvisa.processes.getConvertersTo(dest, keepType=1, checkUpdate=True)[source]¶
Gets the converters which can convert data to destination format.
- Parameters:
destination – tuple (type, format). If a converter is not found directly, parent types are tried.
keepType (boolean) – if True, parent type won’t be tried. Default True.
checkUpdate (boolean) – if True, Brainvisa will check if the converters needs to be reloaded. Default True.
- Returns:
a dict (type, format) ->
NewProcess
class associated to the found converter.
- brainvisa.processes.getDataEditor(source, enableConversion=0, checkUpdate=True, listof=False, index=0, process=None, check_values=False)[source]¶
Gets a data editor (a process that have the role editor) which can open source data for edition (modification). The data editor is returned only if its userLevel is lower than the current userLevel.
- Parameters:
source – a
neuroDiskItems.DiskItem
, a list ofneuroDiskItems.DiskItem
(only the first will be taken into account), a tuple (type, format).enableConversion (boolean) – if True, a data editor that accepts a format in which source can be converted is also accepted. Default False
checkUpdate (boolean) – if True, Brainvisa will check if the editor needs to be reloaded. Default True.
listof (boolean) – If True, we need an editor for a list of data. If there is no specific editor for a list of this type of data, a
ListOfIterationProcess
is created from the associated simple editor. Default False.process (None or NewProcess class or instance) – if specified, specialized viewers having a variable ‘allowed_processes’ which list this process, will be sorted first
check_values (bool) – if True, check if the 1st parameter of each data editor actually accepts the source value. This is not always true because some filtering may happen using some requiredAttribues.
- Returns:
editor
- Return type:
the
NewProcess
class associated to the found editor.
- brainvisa.processes.getDataEditors(source, enableConversion=1, checkUpdate=True, listof=False, process=None, check_values=False)[source]¶
Get data editors
NewProcess
able to edit aneuroDiskItems.DiskItem
source.Data editors are ordered using the distance of
processes.DiskItemConversionInfo
between theneuroDiskItems.DiskItem
source to edit and theneuroDiskItems.DiskItem
source registered for the data editor. The data editors registered with closest sources appear first.- Parameters:
enableConversion (int) – if the source can be converted to be edited. Default 1.
checkUpdate (boolean) – if converters and data editors
NewProcess
must be reloaded when they changed. Default True.listof (boolean) – if the viewers
NewProcess
must be able to edit list ofneuroDiskItems.DiskItem
. Default False.process (None or NewProcess class or instance) – if specified, specialized data editors having a variable ‘allowed_processes’ which list this process, will be sorted first
check_values (bool) – if True, check if the 1st parameter of each data editor actually accepts the source value. This is not always true because some filtering may happen using some requiredAttribues.
- Returns:
a list of data editors
NewProcess
.- Return type:
data editors
- brainvisa.processes.getDefaultListOfDataEditor(source, enableConversion=1, checkUpdate=True, process=None)[source]¶
Get a default data editor for a list of
neuroDiskItems.DiskItem
.- Parameters:
enableConversion (int) – if the source can be converted to be
edited. Default 1. :param boolean checkUpdate: if converters and data editors
NewProcess
must be reloaded when they changed. Default True.- Returns:
Callable Object that can be used to edit a list of
neuroDiskItems.DiskItem
.
- brainvisa.processes.getDefaultListOfProcesses(source, role, enableConversion=1, checkUpdate=True, process=None)[source]¶
Get a default processes able to play the given role for a list of
neuroDiskItems.DiskItem
.- role: str
the role of processes to get (mainly ‘viewer’ or ‘editor)
- enableConversion: int
if the source can be converted to be used. Default 1.
- checkUpdate: boolean
if processes
NewProcess
must be reloaded when they changed. Default True.
- Returns:
Callable Object that can play the given role for a list of
neuroDiskItems.DiskItem
.
- brainvisa.processes.getDefaultListOfViewer(source, enableConversion=1, checkUpdate=True, process=None)[source]¶
Get a default viewer for a list of
neuroDiskItems.DiskItem
.- Parameters:
enableConversion (int) – if the source can be converted to be
visualized. Default 1. :param boolean checkUpdate: if converters and viewers
NewProcess
must be reloaded when they changed. Default True.- Returns:
Callable Object that can be used to visualize a list of
neuroDiskItems.DiskItem
.
- brainvisa.processes.getDiskItemSourceInfo(source)[source]¶
Gets a tuple containing source
neuroDiskItems.DiskItemType
andneuroDiskItems.Format
.- Parameters:
source – a
neuroDiskItems.DiskItem
, a list ofneuroDiskItems.DiskItem
(only the first will be taken into account), a tuple (type, format).
- brainvisa.processes.getHTMLFileName(processId, documentation=None, language=None)[source]¶
Gets the path to the html page corresponding to the documentation of the process in parameter.
- brainvisa.processes.getImporter(source, checkUpdate=True)[source]¶
Gets a importer (a process that have the role importer) which can import data in the database.
- Parameters:
source – a
neuroDiskItems.DiskItem
or a tuple (type, format).checkUpdate (boolean) – if True, Brainvisa will check if the process needs to be reloaded. Default True.
- Returns:
the
NewProcess
class associated to the found process.
- brainvisa.processes.getNearestConverter(source, dest, checkUpdate=True)[source]¶
Gets the nearest converter which can convert data from source to dest.
- Parameters:
source – tuple (type, format). If a converter is not found directly, parent types are tried.
dest – tuple (type, format). If a converter is not found directly, parent types are tried.
checkUpdate (boolean) – if True, Brainvisa will check if the converters needs to be reloaded. Default True.
- Returns:
a tuple ((source_distance, dest_distance), process)
NewProcess
class associated to the found converter.
- brainvisa.processes.getProcdocFileName(processId)[source]¶
Returns the name of the file (.procdoc) that contains the documentation of the process in parameter.
- brainvisa.processes.getProcess(processId, ignoreValidation=False, checkUpdate=True)[source]¶
Gets the class associated to the process id given in parameter.
When the processes are loaded, a new class called
NewProcess
is created for each process. This class inherits fromProcess
and adds an instance counter which is incremented each time a new instance of the process is created.- Parameters:
processId – the id or the name of the process, or a dictionary {‘type’ : ‘iteration|distributed|selection’, ‘children’ : […] } to create an
IterationProcess
, aDistributedProcess
or aSelectionProcess
.ignoreValidation (boolean) – if True the validation function of the process won’t be executed if the process need to be reloaded - default False.
checkUpdate (boolean) – If True, the modification date of the source file of the process will be checked. If the file has been modified since the process loading, it may need to be reloaded. The user will be asked what he wants to do. Default True.
- Returns:
a
NewProcess
class which inherits fromProcess
.
- brainvisa.processes.getProcessFromExecutionNode(node)[source]¶
Gets a process instance corresponding to the given execution node.
- Parameters:
node – a process
ExecutionNode
- Returns:
According to the type of node, it returns:
a
NewProcess
instance if the node isProcessExecutionNode
,an
IterationProcess
if the node is aSerialExecutionNode
a
DistributedProcess
if the node is aParallelExecutionNode
a
SelectionProcess
if the node is aSelectionExecutionNode
.
- brainvisa.processes.getProcessInfo(processId)[source]¶
Gets information about the process whose id is given in parameter.
- Return type:
- brainvisa.processes.getProcessInstance(processIdClassOrInstance, ignoreValidation=False)[source]¶
Gets an instance of the process given in parameter.
- Parameters:
processIdClassOrInstance (a process id, name, class, instance, execution node, or a the name of a file containing a backup copy of a process.) –
ignoreValidation (bool (optional)) – if True, a validation failure will not prevent from building an instance of the process.
- Return type:
an instance of the
NewProcess
class associated to the described process.
- brainvisa.processes.getProcessInstanceFromProcessEvent(event)[source]¶
Gets an instance of a process described in a
brainvisa.history.ProcessExecutionEvent
.- Parameters:
event – a
brainvisa.history.ProcessExecutionEvent
that describes the process: its structure and its parameters.- Returns:
an instance of the
NewProcess
class associated to the described process. Parameters may have been set.
- brainvisa.processes.getProcessesBySource(source, role, enableConversion=1, checkUpdate=True, listof=False, process=None, check_values=False)[source]¶
Get processes
NewProcess
able to play the given role for aneuroDiskItems.DiskItem
source.Processes are ordered using the distance of
processes.DiskItemConversionInfo
between theneuroDiskItems.DiskItem
source to use and theneuroDiskItems.DiskItem
source registered for the process. The processes registered with closest sources appear first.- Parameters:
role (str) – the role of processes to get (mainly ‘viewer’ or ‘editor’)
enableConversion (int) – if the source can be converted to find appropriate processes. Default 1.
checkUpdate (boolean) – if processes
NewProcess
must be reloaded when they changed. Default True.listof (boolean) – if the processes
NewProcess
must be able to use list ofneuroDiskItems.DiskItem
. Default False.process (None or NewProcess class or instance) – if specified, specialized processes having a variable ‘allowed_processes’ which list this process, will be sorted first
check_values (bool) – if True, check if the 1st parameter of each process actually accepts the source value. This is not always true because some filtering may happen using some requiredAttribues.
- Returns:
a list of processes
NewProcess
.- Return type:
processes
- brainvisa.processes.getProcessesBySourceDist(registry, source, enableConversion=1, exactConversionTypeOnly=False, checkUpdate=True)[source]¶
Get processes
NewProcess
able to process aneuroDiskItems.DiskItem
source using a registry.Processes are ordered using the distance of
processes.DiskItemConversionInfo
between theneuroDiskItems.DiskItem
source to process and theneuroDiskItems.DiskItem
source registered for the process. The processes registered with closest sources appear first.- Parameters:
registry (dict) – the registry of
processes.ProcessSet
. Keys
are a tuple containing
neuroDiskItems.DiskItemType
andneuroDiskItems.Format
. Values areprocesses.ProcessSet
. :param int enableConversion: if the source can be converted to be processed. Default 1. :param boolean exactConversionTypeOnly: Specify if the conversion is valid only when converter is registered for the exact destinationneuroDiskItems.DiskItemType
. Default False :param boolean checkUpdate: if processesNewProcess
must be reloaded when they changed. Default True.- Returns:
a list of processes
NewProcess
.
- brainvisa.processes.getViewer(source, enableConversion=1, checkUpdate=True, listof=False, index=0, process=None, check_values=False)[source]¶
Gets a viewer (a process that have the role viewer) which can visualize source data. The viewer is returned only if its userLevel is lower than the current userLevel.
- Parameters:
source – a
neuroDiskItems.DiskItem
, a list ofneuroDiskItems.DiskItem
(only the first will be taken into account), a tuple (type, format).enableConversion (boolean) – if True, a viewer that accepts a format in which source can be converted is also accepted. Default True
checkUpdate (boolean) – if True, Brainvisa will check if the viewer needs to be reloaded. Default True.
listof (boolean) – If True, we need a viewer for a list of data. If there is no specific viewer for a list of this type of data, a
ListOfIterationProcess
is created from the associated simple viewer. Default False.index (int) – index of the viewer to find. Default 0.
process (None or NewProcess class or instance) – if specified, specialized viewers having a variable ‘allowed_processes’ which list this process, will be sorted first
check_values (bool) – if True, check if the 1st parameter of viewer actually accepts the source value. This is not always true because some filtering may happen using some requiredAttribues.
- Returns:
the
NewProcess
class associated to the found viewer or None if not found at the specified index.- Return type:
viewer
- brainvisa.processes.getViewers(source, enableConversion=1, checkUpdate=True, listof=False, process=None, check_values=False)[source]¶
Get viewers
NewProcess
able to visualize aneuroDiskItems.DiskItem
source.Viewers are ordered using the distance of
processes.DiskItemConversionInfo
between theneuroDiskItems.DiskItem
source to visualize and theneuroDiskItems.DiskItem
source registered for the viewer. The viewers registered with closest sources appear first.- Parameters:
enableConversion (int) – if the source can be converted to be visualized. Default 1.
checkUpdate (boolean) – if converters and viewers
NewProcess
must be reloaded when they changed. Default True.listof (boolean) – if the viewers
NewProcess
must be able to display list ofneuroDiskItems.DiskItem
. Default False.process (None or NewProcess class or instance) – if specified, specialized viewers having a variable ‘allowed_processes’ which list this process, will be sorted first
check_values (bool) – if True, check if the 1st parameter of each viewer actually accepts the source value. This is not always true because some filtering may happen using some requiredAttribues.
- Returns:
a list of viewers
NewProcess
.- Return type:
viewers
- brainvisa.processes.initializeProcesses()[source]¶
Intializes the global variables of the module. The current thread is stored as the main thread. A default execution context is created.
- brainvisa.processes.mainThreadActions()[source]¶
Returns an object which allows to pass actions to be executed in the main thread. Its implementation may differ according to the presence of a running graphics event loop, thus the returned object may be an instance of different classes:
soma.qtgui.api.QtThreadCall
,soma.qtgui.api.FakeQtThreadCall
, or even something else.In any case the returned mainthreadactions object has 2 methods, call() and push():
result = mainthreadactions.call(function, *args, **kwargs) #or mainthreadactions.push(function, *args, **kwargs)
- brainvisa.processes.mapValuesToChildrenParameters(destNode, sourceNode, dest, source, value=None, defaultProcess=None, defaultProcessOptions={}, name=None, resultingSize=-1, allow_remove=False)[source]¶
Maps values of parameter sourceNode.*source* (which is a list) to destNode.*dest*. value will receive parameter value when calling this function from a link. If children are fewer than source values and a defaultProcess is given, lacking children are added, with chosen defaultProcessOptions and name if given. Should resultingSize value differ from -1, the resulting number of children will be set to this value. If allow_remove is False (the default), nodes will never be removed even if source list is shorter than the number of nodes.
sourceNode, source, and dest may be lists of parameters (of matching sizes if they are lists) to map. The longest size will determine the number of nodes. destNode should not be a list since it is the child node we are working on.
- brainvisa.processes.pathsplit(path)[source]¶
Returns a tuple corresponding to a recursive call to os.path.split for example on Unix:
pathsplit('toto/titi/tata') == ('toto', 'titi', 'tata') pathsplit('/toto/titi/tata') == ('/', 'toto', 'titi', 'tata')
- brainvisa.processes.procdocToXHTML(procdoc)[source]¶
Converts HTML tags in the content of a .procdoc file to XHTML tags. Checks its syntax.
- brainvisa.processes.readProcdoc(processId)[source]¶
Returns the content of the documentation file (.procdoc) of the process in parameter.
- brainvisa.processes.readProcess(fileName, category=None, ignoreValidation=False, toolbox='brainvisa')[source]¶
Loads a process from its source file. The source file is a python file which defines some variables (signature, name, userLevel) and functions (validation, initialization, execution).
The process is indexed in the global lists of processes so it can be retrieved through the functions
getProcess()
,getProcessInfo()
,getViewer()
, …- Parameters:
fileName (string) – the name of the file containing the source code of the process.
category (string) – category of the process. If None, it is the name of the directory containing the process.
ignoreValidation (boolean) – if True, the validation function of the process won’t be executed.
toolbox (string) – The id of the toolbox containing the process. Defaut is ‘brainvisa’, it indicates that the process is not in a toolbox.
- Returns:
A
NewProcess
class representing the process if no exception is raised during the loading of the process.
A new class derived from
Process
is defined to store the content of the file:- class brainvisa.processes.NewProcess¶
Bases:
Process
All the elements defined in the file are added to the class.
- name¶
Name of the process. If it is not defined in the process file, it is the base name of the file without extension.
- category¶
The category of the process. If it is not given in parameter, it is the name of the directory containing the process file.
- dataDirectory¶
The data directory of the process is a directory near the process file with the same name and the extension .data. It is optional.
- processReloadNotifier¶
A
soma.notification.Notifier
that will notify its observers when the process is reload.
- signature¶
The parameters excepted by the process.
- userLevel¶
Minimum userLevel needed to see the process.
- roles¶
Roles of the process: viewer, converter, editor, impoter.
- execution(self, context)¶
Execution function.
- brainvisa.processes.readProcesses(processesPath)[source]¶
Read all the processes found in toolboxes and in a list of directories. The toolboxes are found with the function
brainvisa.toolboxes.allToolboxes()
.A global object representing a tree of processes is created, it is an instance of the
ProcessTree
- Parameters:
processesPath (list) – list of paths to directories containing processes files.
- brainvisa.processes.registerConverter(source, dest, process_id)[source]¶
Registers converter from source to destination. :param source: tuple (type, format). If a converter is not found directly, parent types are tried. :param dest: tuple (type, format). If a converter is not found directly, parent types are tried. :param proc:
NewProcess
class associated to the converter
- brainvisa.processes.reloadToolboxes()[source]¶
Reloads toolboxes, processes, types, ontology rules, databases. Useful to take into account new files without having to quit and start again Brainvisa.
- brainvisa.processes.runDataEditor(source, context=None, process=None)[source]¶
Searches for a data editor for source data and runs the process. If data editor fail to edit source, tries to get another.
- Parameters:
source – a
neuroDiskItems.DiskItem
or something that enables to find aneuroDiskItems.DiskItem
.context – the
ExecutionContext
. If None, the default context is used.
- Returns:
the result of the execution of the found data editor.
- brainvisa.processes.runProcessBySource(source, role, context=None, process=None, continueOnError=True)[source]¶
Searches for a viewer for source data and runs the process. If viewer fail to display source, tries to get another.
- Parameters:
source – a
neuroDiskItems.DiskItem
or something that enables to find aneuroDiskItems.DiskItem
.context – the
ExecutionContext
. If None, the default context is used.
- Returns:
the result of the execution of the found viewer.
- brainvisa.processes.runViewer(source, context=None, process=None)[source]¶
Searches for a viewer for source data and runs the process. If viewer fail to display source, tries to get another.
- Parameters:
source – a
neuroDiskItems.DiskItem
or something that enables to find aneuroDiskItems.DiskItem
.context – the
ExecutionContext
. If None, the default context is used.
- Returns:
the result of the execution of the found viewer.
- brainvisa.processes.setMainThreadActionsMethod(method)[source]¶
Set the mainThreadActions loop method.
- Parameters:
method (soma.qt_gui.qtThread.FakeQtThreadCall or soma.qt_gui.qtThread.QtThreadCall object) – instance of the thread handler
- brainvisa.processes.updateProcesses()[source]¶
Called when option userLevel has changed. Associated widgets will be updated automatically because they listens for changes.
brainvisa.processing.neuroException¶
The functions are used to display error and warning messages in Brainvisa.
showException()
can be used to display a message describing the last exception that occured in Brainvisa error window or in the console. In the same way, the function showWarning()
can be used to display warning message.
Example
>>> try:
>>> <code that can raise an exception>
>>> except:
>>> neuroException.showException(beforeError="The following error occured when...:")
- class brainvisa.processing.neuroException.DumbHTMLPrinter(formatter)[source]¶
Bases:
HTMLParser
,object
Initialize and reset this instance.
If convert_charrefs is True (the default), all character references are automatically converted to the corresponding Unicode characters.
- class brainvisa.processing.neuroException.HTMLMessage(msg)[source]¶
Bases:
object
This class enables to create an error message in HTML format. Creates an instance of this class to raise an error with an HTML message.
Example: raise RuntimeError( HTMLMessage(“<b>Error …</b>”) )
- brainvisa.processing.neuroException.catch_gui_exception(method)[source]¶
A decorator for Qt5 slots.
Runs the method in a try… except block, and if an exception occurs, show it in the GUI and return. This decorator can be used for PyQt5 slots which do not catch exceptions by default as PyQt4 used to do.
- brainvisa.processing.neuroException.exceptionHTML(beforeError='', afterError='', exceptionInfo=None)[source]¶
Generates an HTML message that describes the given exception with its traceback.
- Parameters:
exceptionInfo (tuple) – (type, value, traceback) describing the exception.
beforeError (string) – Message that will be displayed before the text of the exception.
afterError (string) – Message that will be displayed after the text of the exception.
- Return type:
string
- Returns:
the message in HTML format.
- brainvisa.processing.neuroException.exceptionMessageHTML(exceptionInfo, beforeError='', afterError='')[source]¶
Generates an HTML message that describes the given exception. The traceback of the exception is not included in the message.
- Parameters:
exceptionInfo (tuple) – (type, value, traceback) describing the exception.
beforeError (string) – Message that will be displayed before the text of the exception.
afterError (string) – Message that will be displayed after the text of the exception.
- Return type:
string
- Returns:
the message in HTML format.
- brainvisa.processing.neuroException.exceptionTracebackHTML(exceptionInfo)[source]¶
Generates an HTML message that describes the traceback of the given exception.
- Parameters:
exceptionInfo (tuple) – (type, value, traceback) describing the exception.
- Return type:
string
- Returns:
the message in HTML format.
- brainvisa.processing.neuroException.logException(beforeError='', afterError='', exceptionInfo=None, context=None)[source]¶
Generates two HTML messages to represent the current exception: a short one and a detailed version. The exception is also stored in Brainvisa log file. The detailed message shows the traceback of the exception. The short message is generated with the function
exceptionMessageHTML()
and the detailed one withexceptionTracebackHTML()
.- Parameters:
beforeError (string) – Message that will be displayed before the text of the exception.
afterError (string) – Message that will be displayed after the text of the exception.
exceptionInfo (tuple) – tuple (type, value, traceback) describing the exception. If None,
sys.exc_info()
is used to get the exception.context –
brainvisa.processes.ExecutionContext
that can be used to store the message at the right place in the log file. Indeed, the current log could be the log of a process execution. If None, the default context is used.
- Return type:
- Returns:
A short HTML message and a detailed version of the message.
- brainvisa.processing.neuroException.print_html(message, file=<_io.TextIOWrapper name='<stdout>' mode='w' encoding='utf-8'>, flush=True)[source]¶
Print a HTML message converted to plain text
- brainvisa.processing.neuroException.showException(beforeError='', afterError='', parent=None, gui=None, exceptionInfo=None)[source]¶
Displays an error message describing the last exception that occurred or the exception information given in parameter. The message can be displayed in Brainvisa error window or in the console. The generated message is in HTML format and have a style adapted to error messages (icon, font color).
- Parameters:
beforeError (string) – Message that will be displayed before the text of the exception.
afterError (string) – Message that will be displayed after the text of the exception.
parent – A parent widget for the exception widget. Optional.
gui (boolean) – If True, the graphical interface is used to display the exception. Else, it is displayed in the console. If None, it is displayed with the graphical interface if Brainvisa is in graphical mode.
exceptionInfo (tuple) – tuple (type, value, traceback) describing the exception. If None,
sys.exc_info()
is used to get the exception.
- brainvisa.processing.neuroException.showWarning(message, parent=None, gui=None)[source]¶
Shows a warning message. The message can be displayed in Brainvisa error window or in the console. The generated message is in HTML format and have a style adapted to warning messages (icon, font color).
- Parameters:
message (string) – Warning message that will be displayed.
parent – A parent widget for the exception widget. Optional.
gui (boolean) – If True, the graphical interface is used to display the exception. Else, it is displayed in the console. If None, it is displayed with the graphical interface if Brainvisa is in graphical mode.
brainvisa.processing.neuroLog¶
This module contains the classes for Brainvisa log system.
Brainvisa main log is an instance of LogFile
. It is stored in the global variable brainvisa.configuration.neuroConfig.mainLog
.
This main log is created in the function initializeLog()
.
- Inheritance diagram:
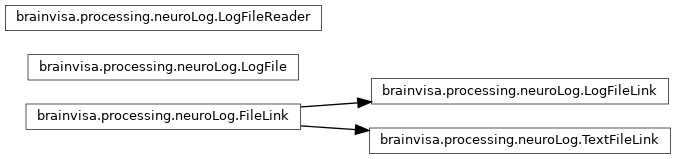
- Classes and functions:
- class brainvisa.processing.neuroLog.FileLink[source]¶
Bases:
object
Base virtual class for a link on a log file.
- class brainvisa.processing.neuroLog.LogFile(fileName, parentLog, lock, file=None, temporary=False)[source]¶
Bases:
object
This class represents Brainvisa log file. This object is structured hierarchically. A LogFile can have a parent log and can be the parent log of other logs.
This hierarchical structure is useful in Brainvisa because several threads may have to add log information at the same time, so we have to use several temporary log files to avoid concurrent access to the same file. So each process have its own LogFile which can have sub logs if the process calls other processes or system commands. The different log files are merged in the main log file when the process ends.
The content of the file is in minf xml format.
The elements written in the file are
Item
objects. They are written through the methodappend()
.- Parameters:
fileName (string) – path to the file where the log information will be written.
parentLog – parent
Logfile
.lock –
threading.RLock()
, a lock to prevent from concurrent access to the file.
- Parent file:
stream on the opened file.
- class Item(what, when=None, html='', children=[], icon=None)[source]¶
Bases:
object
An entry in a log file. It can have a list of children items.
- Parameters:
what (string) – title of the entry
when – date of creation returned by
time.time()
by default.html – the content associated to this item in HTML format. String or
FileLink
.children – sub items: a list of Items or a LogFile (which contains Items)
icon (string) – An icon file associated to this entry.
- class SubTextLog(fileName, parentLog)[source]¶
Bases:
TextFileLink
This class is a kind of leaf in the tree of log files. It cannot be a parent for another log file. It only stores text information.
- Parameters:
fileName (string) – path to the file where the log information will be written.
parentLog – parent
brainvisa.processing.neuroLog.LogFile
.
- append(*args, **kwargs)[source]¶
Writes an
Item
in the current log file. This method can take in parameters aItem
or the parameters needed to create a newItem
.
- expand(force=False)[source]¶
Reads the current log file and the sub logs and items files and merges all their content in a same file.
- subLog(fileName=None)[source]¶
Creates a sub log, that is to say a new
LogFile
which have this log as parent log.- Parameters:
fileName (string) – name of the file where log information will be written. If None, the sublog is associated to a new temporary file created with
brainvisa.data.temporary.TemporaryFileManager.new()
.- Return type:
- Returns:
The new sub log.
- subTextLog(fileName=None)[source]¶
Creates a
SubTextLog
as a child of the current log. The sub log have the current log as parent log. This type of sub log is used for example to store the output of a system command.- Parameters:
fileName (string) – name of the file where log information will be written. If None, the sublog is associated to a new temporary file created with
brainvisa.data.temporary.TemporaryFileManager.new()
.- Return type:
- Returns:
The new sub log.
- class brainvisa.processing.neuroLog.LogFileLink(fileName)[source]¶
Bases:
FileLink
This class represents a link on the file associated to a
LogFile
.
- class brainvisa.processing.neuroLog.LogFileReader(source)[source]¶
Bases:
object
This objects enables to read
LogFile
Logfile.Item
from a filename.
- class brainvisa.processing.neuroLog.TextFileLink(fileName)[source]¶
Bases:
FileLink
This class represents a link on the file associated to a
SubTextLog
.- Parameters:
fileName (string) – name of the file
- brainvisa.processing.neuroLog.closeMainLog()[source]¶
Closes Brainvisa main log. The log file content is expanded and the file is compressed with
gzip.GzipFile
.
- brainvisa.processing.neuroLog.expandedCopy(source, destFileName, destFile=None)[source]¶
Returns a copy of the source log file with all its items expanded (file links replaced by the content of the file).
- brainvisa.processing.neuroLog.expandedReader(source)[source]¶
Generator on the
Item
of the source file. Each item is expanded, that is to say each file link that they contains is also read.
- brainvisa.processing.neuroLog.initializeLog()[source]¶
Creates Brainvisa main log as an instance of
LogFile
which is stored in the variablebrainvisa.configuration.neuroConfig.mainLog
. The associated file isbrainvisa.configuration.neuroConfig.logFileName
.
brainvisa.processing.capsul_process¶
Specialized Process class to link with CAPSUL processes and pipelines.
the aim is to allow using a Capsul process/pipeline as an Axon process (or at least, ease it). Such a process would look like the following:
from brainvisa.processes import *
from brainvisa.processing import capsul_process
name = 'A Capusl process ported to Axon'
userLevel = 0
base_class = capsul_process.CapsulProcess
capsul_process = 'morphologist.capsul.morphologist.Morphologist'
Explanation:
The process should inherit the CapsulProcess class (itself inheriting the standard Process). To do so, it declares the “base_class” variable to this CapsulProcess class type.
Then it should instantiate the appropriate Capsul process. This is done simlply by setting the variable capsul_process
(or alternately by overloading the setup_capsul_process() method, which should instantiate the Capsul process and set it into the Axon proxy process).
The underlying Capsul process traits will be exported to the Axon signature automatically. This behaviour can be avoided or altered by overloading the initialize() method, which we did not define in the above example.
The process also does not have an execution()
function. This is normal: CapsulProcess
already defines an executionWorkflow()
method which will generate a Soma-Workflow workflow which will integrate in the process or parent pipeline (or iteration) workflow.
nipype interfaces
As Capsul processes can be built directly from nipype interfaces, any nipype interface can be used in Axon wrappings here.
Pipeline design directly using Capsul nodes¶
In an Axon pipeline, it is now also possible (since Axon 4.6.2) to use directly a Capsul process in an Axon pipeline. In the pieline initialization() method, the pipeline definition code can add nodes which are actually Capsul processes (or pipelines). Capsul processes identifier should start with capsul://
:
from brainvisa.processes import *
name = 'An Axon pipeline'
userLevel = 0
signature = Signature(
'skeleton', ReadDiskItem('Raw T1 MRI', 'aims readable volume formats')
)
def initialization(self):
eNode = SerialExecutionNode(self.name, parameterized=self)
# add an Axon process node
eNode.addChild('SulciSkeleton',
ProcessExecutionNode('sulciskeleton', optional=1)
# add a Capsul process node
eNode.addChild(
'SulciLabeling',
ProcessExecutionNode(
'capsul://deepsulci.sulci_labeling.capsul.labeling',
optional=1))
# link parameters
eNode.addDoubleLink('skeleton', 'SulciSkeleton.skeleton')
eNode.addDoubleLink('SulciSkeleton.skeleton', 'SulciLabeling.skeleton')
# ...
self.setExecutionNode(eNode)
Note that using this syntax, a Capsul process wihch does not have an explicit Axon wraping will be automatically wraped in a new CapsulProcess subclass. This way any Capsul process can be used on-the-fly without needing to define their correspondance in Axon.
However if a wrapper process is already defined in Axon (either to make it visible in Axon interface, or because it needs some customization), then the wrapper process will be found, and used. In this situation the pipeline developer thus can either use the Axon wrapper (ProcessExecutionNode('sulci_deep_labeling')
here) or the Capsul process identifier (ProcessExecutionNode('capsul://deepsulci.sulci_labeling.capsul.labeling')
here). The result will be the same.
Note that nipype interfaces can also be used directly, the exact same way:
eNode.addChild(
'Smoothing',
ProcessExecutionNode(
'capsul://nipype.interfaces.spm.smooth', optional=1))
Process / pipeline parameters completion¶
Capsul and Axon are using parameters completions systems with very different designs (actually Capsul was written partly to overcome Axon’s completion limitaions) and thus are difficult to completely integrate. However some things could be done.
A Capsul process will get completion using Capsul’s completion system. Thus it should have a completion defined (throug a File Organization Model typically). The completion system in Capsul defines some attributes.
The attributes defined in Capsul will be parsed in an Axon process, and using attributes and file format extensions of process parameters, Axon will try to guess matching DiskItem types in Axon (Capsul has no types).
For this to work, matching DiskItem types must be also defined in Axon, and they should be described in a files hierarchy. Thus, some things must be defined more or less twice…
What to do when it does not work
In some situations a matching type in Axon will not be found, either because it does not exist in Axon, or because it is declared in a different, complex way. And sometimes a type will be found but is not the one which should be picked, when several possible types are available.
It is possible to force the parameters types on Axon side, by overloading them in the axon process. To do this, it is possible to define a signature (as in a regular Axon process) which only declares the parameters that need to have their type forced.
For instance if a capsul process has 3 parameters, param_a, param_b, param_c, and we need to assign manually a type to param_b, we would write:
from brainvisa.processes import *
from brainvisa.processing import capsul_process
name = 'A Capusl process ported to Axon'
userLevel = 0
base_class = capsul_process.CapsulProcess
capsul_process = 'my_processes.MyProcess'
signature = Signature(
'param_b', ReadDiskItem('Raw T1 MRI', 'aims readable volume formats'),
)
In this situation the types of param_a and param_c will be guessed, and param_b will be the one from the declared signature. This signature declaration is optional in a wraped Capsul process, of course, when all types can be guessed.
Iterations¶
Capsul has its own iteration system, using iteration nodes in pipelines. Thus Capsul processes are iterated in the Capsul way. For the user it looks differently: parameters are replaced by lists, istead of duplicating the process to be iterated. The GUI is thus more concise and more efficient, and parameters completion is done in Capsul, which is also much more efficient than the Axon way (Capsul completion time is linear with the number of parameters to be completed, where Axon also slows down with the size of the database).
Completion time and bottlenecks¶
The first time a Capsul process is opened, the FOM completion system is initialized (technically the auto-fom mode is used and and all FOMs are read), which takes a few seconds (up to 10s). Later uses will not have this overhead.
Capsul completion system, although not providing immeditate answers, is much more efficient than Axon’s. Typically to perform completion on 1000 iterations over a process of about 5-6 parameters,
Pure Capsul: about 2-3 seconds.
Axon: about 1 minute
Capsul process in Axon: about the same (1 minute) as Axon, because the long part here is the validation of file parameters (checking that files exist, are valid etc), which is always done in Axon whatever the process type.
For a complex pipeline, for instance the Morphologist pipeline, 50 iterations over it will take
Pure Capsul: about 6 seconds
Axon: about 3 minutes and 30 seconds
Capsul process in Axon: about 35 seconds. This is faster than the Axon one because all pipeline internals (nodes parameters) are not exported in Axon.
The specialized “Morphologist Capsul iteration”, which hides the Capsul process inside and does not expose its parameters in Axon, will merely show any completion latency, because completion is delayed to runtime workflow preparation. It will run as fast as the Capsul version.
Process documentation¶
Capsul processes have their documentation conventions: docs are normally in the process docstrings, and are normally written in Sphinx / RestructuredText markup language. A Capsul process in Axon will be able to automatically retreive its Capsul documentation, and convert it into HTML as expected in Axon. The conversion will use pandoc if available to convert the RST markup of Capsul into HTML (which is still not complete Sphinx but easier to use), and the result will be used to generate the process documentation when the generateDocumentation
process is used. Process developpers should not edit the process documentation is Axon though, because then a .procdoc
file would be written and would be used afterwards, therefore the docs will be duplicated and may get out of sync when updated.
See also CAPSUL: what are the plans ?
- class brainvisa.processing.capsul_process.CapsulProcess[source]¶
Bases:
Process
Specialized Process to link with a CAPSUL process or pipeline.
See the
brainvisa.processing.capsul_process
doc for details.- classmethod axon_process_from_capsul_module(process, context=None, pre_signature=None)[source]¶
Create an Axon process from a capsul process instance, class, or module definition (‘morphologist.capsul.morphologist’).
A registry of capsul:axon process classes is maintained
May use build_from_instanc() at lower-level. The main differences with build_from_instance() are:
build_from_instance creates a new process class unconditionally each time it is used
build_from_instance will not check if a process wraping class is defined in axon processes
it will not reuse a cache of process classes
- classmethod build_from_instance(process, name=None, category=None, pre_signature=None, cache=True)[source]¶
Build an Axon process instance from a Capsul process instance, on-the-fly, without an associated module file
- executionWorkflow(context=None)[source]¶
Build the workflow for execution. The workflow will be integrated in the parent pipeline workflow, if any.
StudyConfig options are handled to support local or remote execution, file transfers / translations and other specific stuff.
FOM completion is not performed yet.
- forbid_completion(params, forbid=True)[source]¶
Forbids (blocks) Capsul completion system for the selected parameters. This allows to replace Capsul completion by Axon links when needed (this is more or less the equivalent of unplugging internal links in Axon processes when inserting them in pipelines).
- init_study_config(context=None)[source]¶
Build a Capsul StudyConfig object if not present in the context, set it up, and return it
- initialization()[source]¶
This specialized initialization() method sets up a default signature for the process, duplicating every user trait of the underlying CAPSUL process.
As some parameter types and links will not be correctly translated, it is possible to prevent this automatic behaviour, and to setup manually a new signature, by overloading the initialization() method.
In such a case, the process designer will also probably have to overload the propagate_parameters_to_capsul() method to setup the underlying Capsul process parameters from the Axon one, since there will not be a direct correspondance any longer.
- propagate_parameters_to_capsul()[source]¶
Set the underlying Capsul process parameters values from the Axon process (self) parameters values.
This method will be called before execution to build the workflow.
By default, it assumes a direct correspondance between Axon and Capsul processes parameters, so it will just copy all parameters values. If the initialization() method has been specialized in a particular process, this direct correspondance will likely be broken, so this method should also be overloaded.
- setup_capsul_process()[source]¶
This method is in charge of instantiating the appropriate CAPSUL process or pipeline, and setting it into the Axon process (self), using the set_capsul_process() method.
It may be overloaded by children processes, but the default implementation looks for a variable “capsul_process” in the process source file which provides the Capsul module/process name (as a string), for instance:
capsul_process = "morphologist.capsul.axon.t1biascorrection.T1BiasCorrection"
This is basically the only thing the process must do.
brainvisa.processing.process_based_viewer¶
Specialized viewer process, working with an underlying process.
New in Axon 4.6.
This convenience class is a way to define a viewer process which is linked to another process (or pipeline). A viewer attached to a process will be able to access the reference process parameters in order to build a display using the exact same parameters values. This can avoid ambiguities.
Such a viewer will get an additional attribute, ‘reference_process’, when executing. It can (should) be specialized to work witn one or several processes, which are specified in its ‘allowed_processes’ attribute. The viewer will only be triggered when displaying a parameter of these specific processes. The viewer still needs a signature with a main input parameter, which should match the desired process parameter.
To use it, a specialized viewer process should import this brainvisa.processing.process_based_viewer module, and declare a ‘base_class’ attribute with the value of the ProcessBasedViewer class
Here is an example of a specialized viewer process, using this mechanism:
from brainvisa.processes import ReadDiskItem
from brainvisa.processing.process_based_viewer import ProcessBasedViewer
name = 'Anatomist view bias correction, Morphologist pipeline variant'
base_class = ProcessBasedViewer
allowed_processes = ['morphologist']
signature = Signature(
'input', ReadDiskItem('T1 MRI Bias Corrected',
'anatomist volume formats'),
)
def execution(self, context):
# run the regular viewer using custom parameters
viewer = getProcessInstance('AnatomistShowBiasCorrection')
if not hasattr(self, 'reference_process'):
# fallback to regular behavior
return context.runProcess(viewer, self.input)
# get t1mri and histo_analysis from morphologist pipeline instance
t1mri = self.reference_process.t1mr1
histo_analysis = self.reference_process.histo_analysis
return context.runProcess(
viewer, mri_corrected=self.input,
t1mri=t1mri, histo_analysis=histo_analysis)
Technically, the ProcessBasedViewer class merely delclares some default attribute values for the process:
reference_process = None
roles = ('viewer', )
userLevel = 3
allowed_processes = []
A viewer linked to a process could directly delclare these attibutes instead of inheriting the ProcessBasedViewer class - it is also a matter of code clarity. The viewer mechanism does not check inheritance, but tests the presence of these attributes in the viewer class. A specialized viewer can of course overwrite these attributes, and actually should, at least for the ‘allowed_processes’ variable.
Note that the default roles, ‘viewer’ can be overwriten, typically to use the same mechanism for an editor.
allowed_processes alternative
In some cases allowed processes may not be a fixed list. To handle this situation, ‘allowed_processes’ may be a function instead of a list. This function will be called with a process as argument, and should return a boolean value to tell if the process is accepted for the viewer to work with it. Typically, it can test if the process is part of a specific pipeline:
def allowed_processes(process):
if process.id() == 'morphologist':
return True
pipeline = process.parent_pipeline()
return pipeline is not None and pipeline.id() == 'morphologist'
- class brainvisa.processing.process_based_viewer.ProcessBasedViewer[source]¶
Bases:
Process
Specialized viewer process, working with an underlying process.
See the
brainvisa.processing.process_based_viewer
doc for details.
brainvisa.processing.axon_fso_to_fom¶
- class brainvisa.processing.axon_fso_to_fom.AxonFsoToFom(init_fom_def={}, formats_fom={})[source]¶
Bases:
object
Converter for Axon hierarchies (File System Ontologies) to CAPSUL/Soma-Base FOM (File Organization Model).
Converts parameters for a given process according to rules taken from actual data: a main input data is used for Axon completion, then all parameters are analyzed and converted to FOM entries.
- Parameters:
init_fom_def (dict or (preferably) collections.OrderedDict) – FOM to be completed. An existing one may be used, otherwise a new FOM dictionary is created.
formats_fom (dict or collections.OrderedDict) – Formats and formats lists definitions to be used. They are expected to match Axon formats and formats lists definitions.
- fso_to_fom(proc_name, node_name, data)[source]¶
Transform a process or pipeline parameters into FOM rules. This is the main function in the class.
Subprocesses of a pipeline will be added to the FOM too.
- Parameters:
proc_name (string) – identifier of the Axon process
node_name (string) – name to be used in the FOM
data (string) – input data (file name) for the first input param opf the process. It will be used to perform Axon completion, then to get values and patterns for all parameters. Thus it must be a valid input data, existing in an Axon database.
- Returns:
new_fom_def (collections.OrderedDict) – new FOM definition (also found in self.current_fom_def)
default_atts (dict) – attributes which have default values. Also adde in the FOM, in the “attribute_definitions” section.
- brainvisa.processing.axon_fso_to_fom.fso_to_fom_main(argv)[source]¶
Main FSO hierarchy to FOM conversion for one or several processes. Contains an argument parser for the __main__ function.
- brainvisa.processing.axon_fso_to_fom.ordered_dump(data, stream=None, Dumper=<class 'yaml.dumper.Dumper'>, **kwds)[source]¶
http://stackoverflow.com/questions/5121931/in-python-how-can-you-load-yaml-mappings-as-ordereddicts
- brainvisa.processing.axon_fso_to_fom.ordered_load(stream, Loader=<class 'yaml.loader.Loader'>, object_pairs_hook=<class 'collections.OrderedDict'>)[source]¶
http://stackoverflow.com/questions/5121931/in-python-how-can-you-load-yaml-mappings-as-ordereddicts